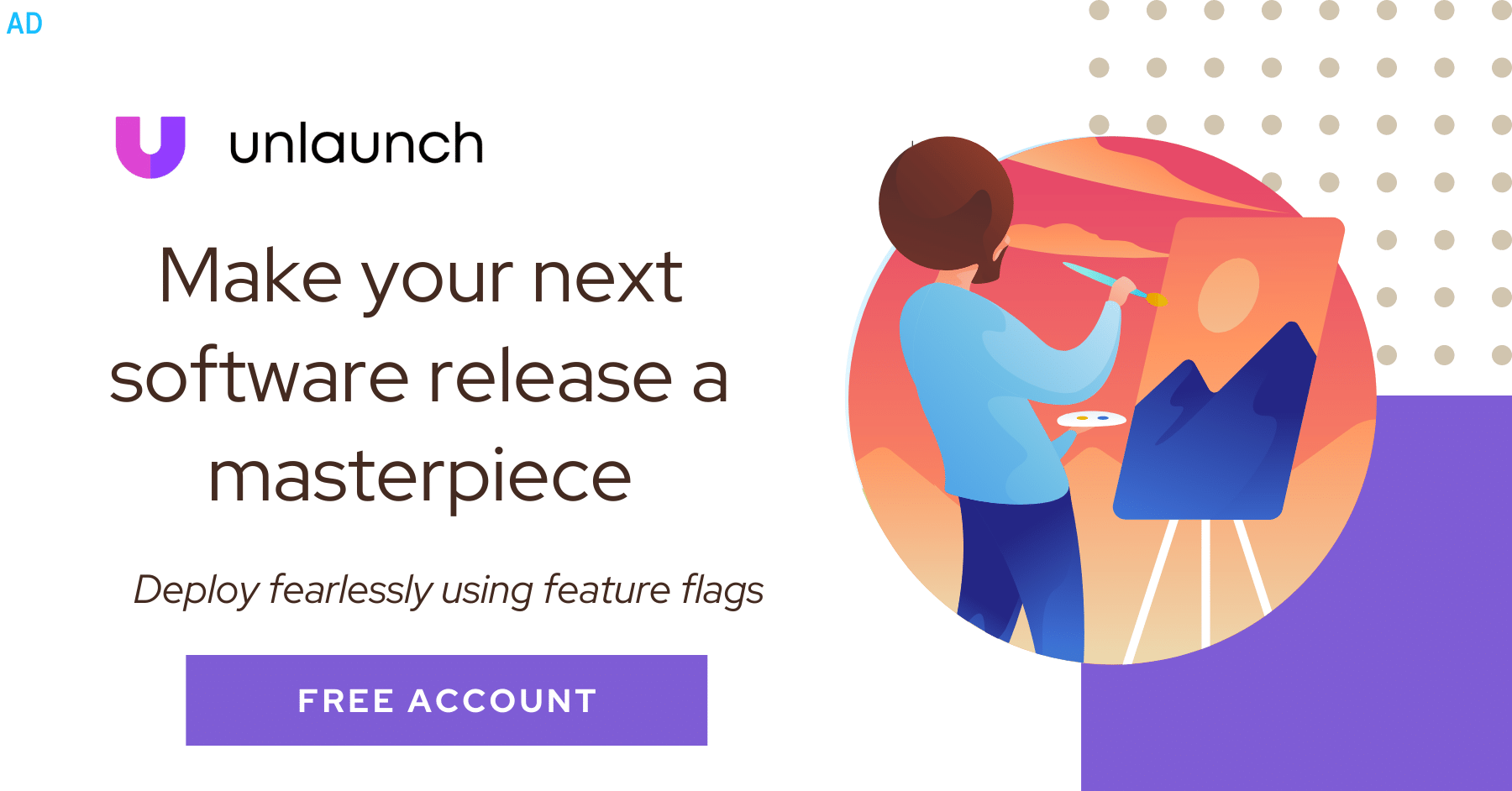
All strings in Java are represented using the String
class. This also includes string literals like “xyz”, which are implemented as instances of the String
class. The length of a string is the total number of characters that it contains (or Unicode code units as we’ll see later.)
The String
class contains many useful string methods. To find the length of a string in Java, we can use the length()
method of the String
class. This method is called on the string object whose length you want to find. It takes no parameters and returns the number of characters (length) as an int
.
In the example below, we are calling the length
method on a string literal, “java”. Because string literals are represented as instances of the String
class, this works:
"java".length(); // 4
String.length() Method Syntax
public int length()
length()
will return the length of the string. The length is equal to the number of characters, or Unicode code units in the string.length()
counts all characters (char
values in a string) including whitespace characters e.g. new line, tabs, etc.- The maximum length of a string is bounded by an
int
which is2^31 - 1
. In other words, a string can have a maximum of 2 billion characters.
Example
In the following example, we call the length
method on a String
object. (In other words, an instance of the String
class)
String domainName = "www.codeahoy.com"; // 16 characters
int length = domainName.length();
System.out.println("the length is: " + length);
Output
the length is: 16
String.length() and Unicode characters
String.length()
method is generally safe for bigger unicode characters. Let’s take a look at an example:
String fireEmoji = "\uD83D\uDD25"; // 🔥
int length = fireEmoji.length();
System.out.println("String.length() is: " + fireEmoji.length());
System.out.println("String.codePointCount() is: " + fireEmoji.codePointCount(0, fireEmoji.length()));
Output
String.length() is: 2
String.codePointCount() is: 1
In the example above, String.length()
returns 2 because the emoji is represented as two char
values (unpaired surrogates). But the String.codePointUnit returns 1 because it counts unpaired surrogates as 1 character. But for many use cases in practice, String.length()
is sufficient.
Here’s the complete example:
public class StringLength {
public static void main(String[] args) {
String domainName = "www.codeahoy.com";
int length = domainName.length();
System.out.println("String.length() is: " + length);
String fireEmoji = "\uD83D\uDD25"; // 🔥
length = fireEmoji.length();
System.out.println("String.length() of " + fireEmoji + " is: " + fireEmoji.length());
System.out.println("String.codePointCount() of " + fireEmoji + " is: " + fireEmoji.codePointCount(0, fireEmoji.length()));
}
}
Output
String.length() is: 16
String.length() of 🔥 is: 2
String.codePointCount() of 🔥 is: 1