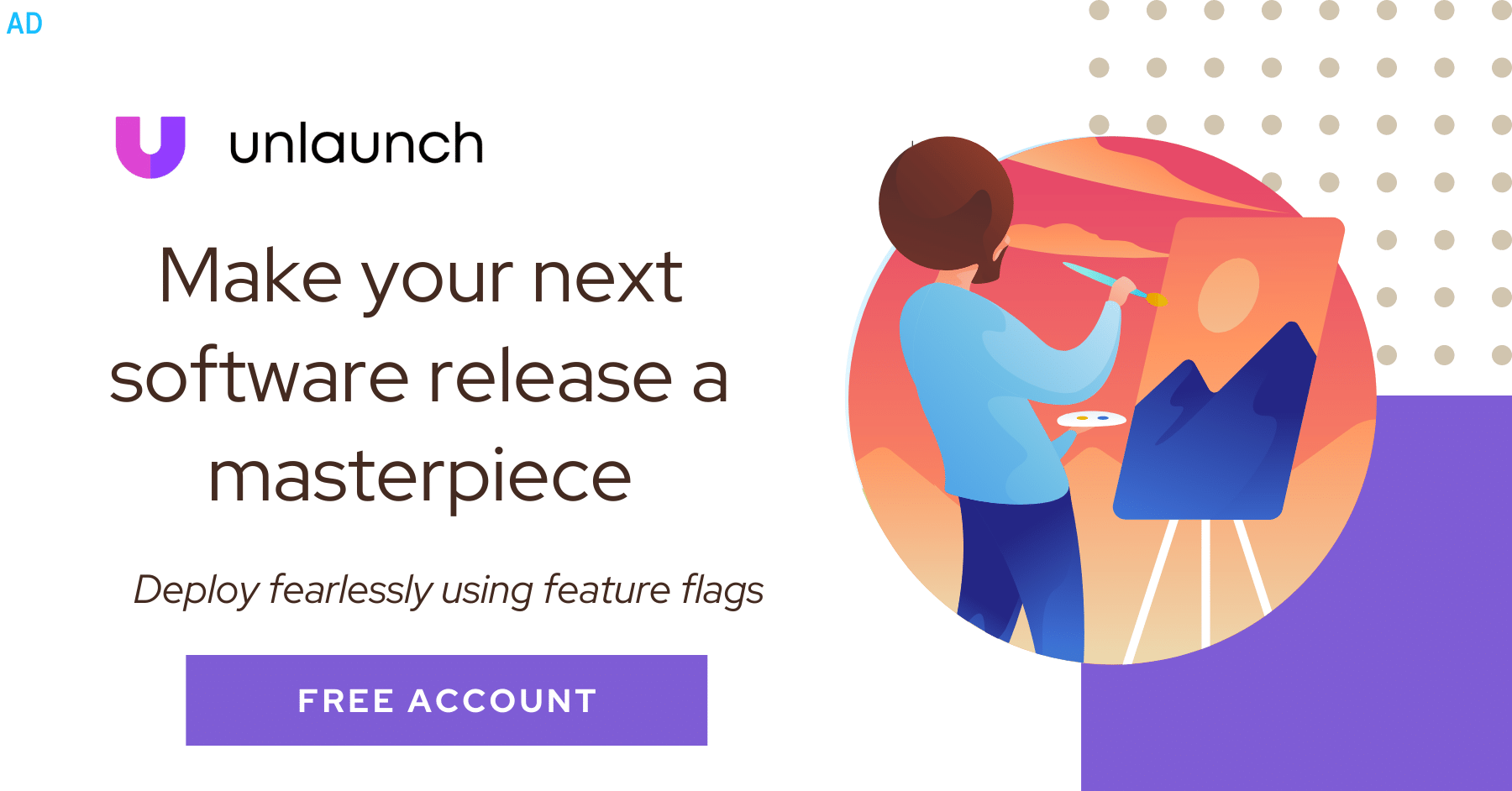
In this tutorial, we’ll see how to convert the primitive char
data type and its wrapper class Character string in Java. We’ll cover three different techniques to convert.
1. String.valueOf(…)
This one is my favorite because it is easy to remember and pretty handy once you understand it: valueOf(...)
are overloaded static methods for data conversion. One such method, String.valueOf(char c), takes char as argument and returns its string representation.
This method should be your preferred way of converting Characters to Strings. Let’s take a look at an example.
package com.codeahoy.ex;
public class Main {
public static void main(String[] args) {
Character c = 'U';
String str = String.valueOf(c);
System.out.println(str);
}
}
Output:
U
As a side note, other overloaded variations of this method can be used to convert integer, double, etc. to string.
- String.valueOf(boolean b) => returns string representation of the boolean argument e.g.
true
orfalse
. - String.valueOf(int i) => returns string representation of the int argument.
- etc.
2. Character.toString()
Character class overrides the toString() method to provide a convenient way of converting Characters to Strings. Here’s an example.
package com.codeahoy.ex;
public class Main {
public static void main(String[] args) {
Character c = 'U';
// Do the conversion using toString()
String str = c.toString();
System.out.println(str);
}
}
Output:
U
3. Coversion by string concatenation
The concatenation (+
) operator is normally used to concatenate two Strings in Java. E.g.
str = s1 + s2
Concatenation operator still works if one of the arguments is of char type and the compiler will type cast automatically. Here’s an example.
package com.codeahoy.ex;
public class Main {
public static void main(String[] args) {
Character c = 'A';
// Conversion using + operator
String str = "" + c;
System.out.println(str);
}
}
Output:
A
That’s all. Until next time.