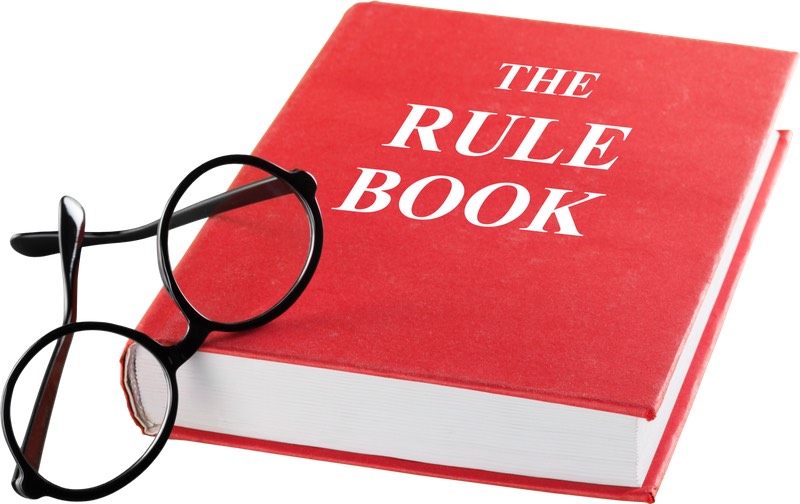
Coding standards are a set of guidelines, best practices, programming styles and conventions that developers adhere to when writing source code for a project. All big software companies have them. Here are few guidelines from the ‘Linux kernel coding style’:
a. Tabs are 8 characters, and thus indentations are also 8 characters.
b. The limit on the length of lines is 80 columns and this is a strongly preferred limit.
c. The preferred form for allocating a zeroed array is the following:
p = kcalloc(n, sizeof(...), ...);
Both forms check for overflow on the allocation size n * sizeof(…), and return NULL if that occurred.
Recently, I came across a blog post from Richard Rodger. In ‘Why I Have Given Up on Coding Standards’, he writes:
Every developer knows you should have a one, exact, coding standard in your company. Every developer also knows you have to fight to get your rules into the company standard. Every developer secretly despairs when starting a new job, afraid of the crazy coding standard some power-mad architect has dictated.
It’s better to throw coding standards out and allow free expression. The small win you get from increased conformity does not move the needle. Coding standards are technical ass-covering.
Oh boy. While I disagree with Richard that coding standards should be abandoned, I share his pain. I briefly worked with a nut job of a “senior developer” who came in as the lead for a project we’d been working on for 6 months. He was an academic who had just finished his PhD and had little experience working on real world projects. He spent first couple of weeks writing “coding standards” in total isolation like he was some kind of a God and we were lowly beings who just weren’t good enough. His coding standards document was full of his personal opinions and promoted some insane form of coding style. The control freak demanded that we update the source code we had already written to reflect his standards. I have never witnessed team morale hit rock bottom so fast. Needless to say, I had a very brief stay at that job.
Another example that I can think of: a manager who insisted on being part of every major code review. During the reviews, he would flag formatting issues, that were almost always a matter of his own preference, as “errors”. The worst part of the story is that he hadn’t written down his coding standards anywhere! I guess he thought developers would learn his style through osmosis. Sometimes, it felt as if he made up rules on the fly. As I mentioned in my post on conducting effective code reviews, it is useless arguing over formatting issues if you don’t have coding standards.
The point is that coding standards are often misunderstood by naive managers and control freaks who misuse them in one way or the other such that it achieves nothing (no one follows them) or causes friction within the team and hurts morale. Many software developers become bitter and start hating coding standards. Coding standards aren’t the problem. Like any other tool, they become harmful when used incorrectly or in the wrong hands. Coding standards that suck have the following attributes:
- full of author’s own opinions and personal coding style. Coding standards are not personal agendas.
- huge focus on style and formatting issues and is often vague.
- recommendations disguised as standards. I have made this mistake. Standards must be treated like rules and hence must be enforceable.
Good software developers and architects understand that coding style is very personal varies from individual to individual. They write coding standards that respect developers’ freedom and allow them to express themselves. They do not attempt to mechanize the whole process, rather they focus on a few well-known practices that are widely accepted or plain common sense. And before any standard is put into practice, they get buy-in from the team, if the team wasn’t already involved in formulating the standards. Here are few examples of good coding standards related to formatting:
- No more than one statement per line.
- Line length should not exceed 80 or 100 characters.
- Test class must start with the name of the class they are testing followed by ‘Test’. E.g.
ServerConfigurationTest
. - One character variable names should only be used in loops or for temporary variables.
All of these could be easily justified in a black-and-white manner without the enforcer appearing like a dictator. On the other extreme, here are some so called “standards” that will rub developers the wrong way and prompt un-necessary debates:
- Class names must not end in
-er
. [Personal Opinion] - Don’t use
static
fields or methods. [Personal Opinion] - Aim for low Cohesion and High Coupling. [Recommendation. Cannot be enforced.]
- Use Test Driven Development. [Recommendation. Cannot be enforced.]
There is a grey area between common sense guidelines and personal preferences such as whether to put braces on the same line or the next. Standardize if you must, but try to keep items in the grey area (generally formatting issues) to a bare minimum.
Effective Coding Standards
Let’s ask the question: why exactly do we need coding standards and what benefits do they offer? Most articles I found online draw a direct relationship between coding standards and software maintainability. While there is absolutely no doubt that source code that adheres to good standards is more readable and reflects harmony, there is another side of coding standards which is often overlooked at the expense of too much attention on aesthetics. Effective coding standards focus on techniques that highlight problems and make bugs stand-out and visible to everyone. Joel said it better in 2005:
Look for coding conventions that make wrong code look wrong.
In Java programming, having the following standards will help catch bugs early on and increase software quality:
- Whenever your override
equals()
method, you must also override thehashCode()
method. - Do not compare strings using
==
or!=
. - Do not ignore exceptions that you caught.
- Do not catch broad exception classes like
Exception
orRuntimeException
.
To recap, effective coding standards:
- are short, simple and concise rules. They do not attempt to cover and processify everything and leave plenty of room for developers to exercise their own creativity.
- strike the right balance between formatting issues and issues that “make the wrong code look wrong”.
- are black and white instead of vague suggestions or recommendations.
Coding standards, when used for the right reasons and in the right manner, offer many benefits. They make source code more readable and the software project more maintainable. They also help catch bugs and mistakes that are disguised as seemingly harmless code. While they might not catch all possible bugs, I’ll take something over nothing any day.
Automate the Process of Checking Code Standards
Once you have effective coding standards, you should automate the process of checking source code’s adherence to standards. It will save a lot of time in peer reviews and catch everything that humans might miss. There is an abundance of style checking tools available for all major programming languages. For our Java projects, we use a popular tool called Checkstyle which checks source code for style and design problems. It provides several Checks and by default, checks code against Sun’s conventions. Or you could choose Google’s coding standards, which I recommend. If you use IntelliJ IDEA (also highly recommended), there’s a checkstyle plugin that shows results right inside the IDE.
If you are starting fresh and looking for coding standards, start by searching online for well-known standards for your programming language. Start small and remember that there is more than one right way to style the code. There might already be a standard available that you could borrow. For Java, I personally like Google’s coding standards that I adopted with slight modifications to indentation rules. Google also have coding standards for many other languages. Your coding standards should check for both style issues and design problems. Once you have standards, make sure that they are adopted by the team and automated. However, code that deviates from standards shouldn’t be considered erroneous. It should simply be marked as out of compliance and deviations must be reviewed and fixed.
Comments (7)
zakius
oh how I hate when someone tells me where to put my braces
keeping them in the same line just hurts my eyes and reduces productivity, especially when day was hard, but for some reason (probably something as ‘important’ as shortening on-paper code listings, while greatly reducing their readability…) many valued programmers, sometimes even gurus enforce this style and are ready to start a war to keep it their way
come on, you can change this to your liking with single hotkey, let me write how I like, and if you can’t review code like this then you can format it yourself when needed, seriously
Umer Mansoor
Yup. Modern IDE’s offer customizations that easily could reorganize code to a format I’m comfortable reading with just a few keystrokes or clicks.
>oh how I hate when someone tells me where to put my braces
I won’t as long as you promise to stay away from the GNU style indents :)(https://en.wikipedia.org/wi….
Christof Damian
I am generally for coding standards. Ideally there should be one set by the creators of the language already (I think go, Python and PHP are pretty good here), or you choose a popular one and adapt it for your need.
What I am dead against is hard enforcement of the standard. I worked at one company where they had a pre-merge check that didn’t allow you to push any code standard violations. This is fine until you change the standard a little bit, and this happened a lot. Once it is changed everyone working on files from before this change had to adapt it to the new style. This made it very difficult to read the diffs and at times introduce bigger problems because refactoring of methods was required.
Umer Mansoor
I have no issues in standards being checked as part of the build process, but deviations from standards shouldn’t be flagged as build errors (unless of course you are writing software to control a nuclear reactor). The report should be used as a compliance check. Some developers I have worked with keep their code in compliance from day one. Others would fix their code in the later stages typically all at once.
$mike cremer
I agree with the main thrust, that coding standards can help to make code readable, might help to highlight dubious or buggy code, and should be (machine) enforceable…
…but at the same time, I do not agree that rules can be neatly divided into “fact” and “opinion.”
In fact, I would go so far as to say the reason we have so many conflicting standards is precisely because we cannot agree on what is fact and what is opinion. And that’s OK.
As a case study, I give you “Miss Manners’ Guide to C++”, which eventually was published as “Taligent’s Guide to Designing Programs: Well-Mannered Object-Oriented Design in C++” [http://www.amazon.com/Talig…]
That document started as a simple set of do’s and don’ts. It was, as the OP might have put it, “full of author’s own opinions and personal coding style.” But it was also the agreed-upon style for the project (“Pink”) and had utility.
Another classic example is Hungarian Notation. I suspect you would find a number of people passionately supporting and others vehemently denying its usefulness.
For this reason, maybe calling them “coding styles” may be nearer the truth.
C.F.
Checkstyle, findbugs, PMD, etc. are all awesome. I’ve found thread-related bugs in Java thanks to one of them, and it saved me tons of time (starting threads in constructors yields to non-deterministic behavior across Java platforms). There are some super-strict linters for JavaScript, too, which I’m keen on using because they save time (catch the gotchas before the interpreter does…).
But the stylistic issues like tabs, spaces and braces? Bikeshedding… If the team is focused on quality and doing good code, I can rise above that level. There are usually options for such things in IDEs and we have so many other details to focus our energy on.
Simon Platten
My own coding standard has evolved since the early 90’s today I believe its robust and the correct way to do things but I would say that. I still see large companies with large development teams with no coding standards at all and senior employees in those companies reject standards that could improve the readability and ease for other developers to read the same source code without spending days or longer cross referencing every line of code. I intend now to put down in print the standards that I use and would love to hear from anyone interested in publishing the standard. The same standard applies to C, C++ and Java and could apply to other languages.
Software developer are a egotistical bunch, every developer thinks his own opinion is the only one that counts which is why introducing a standard can be hard work.