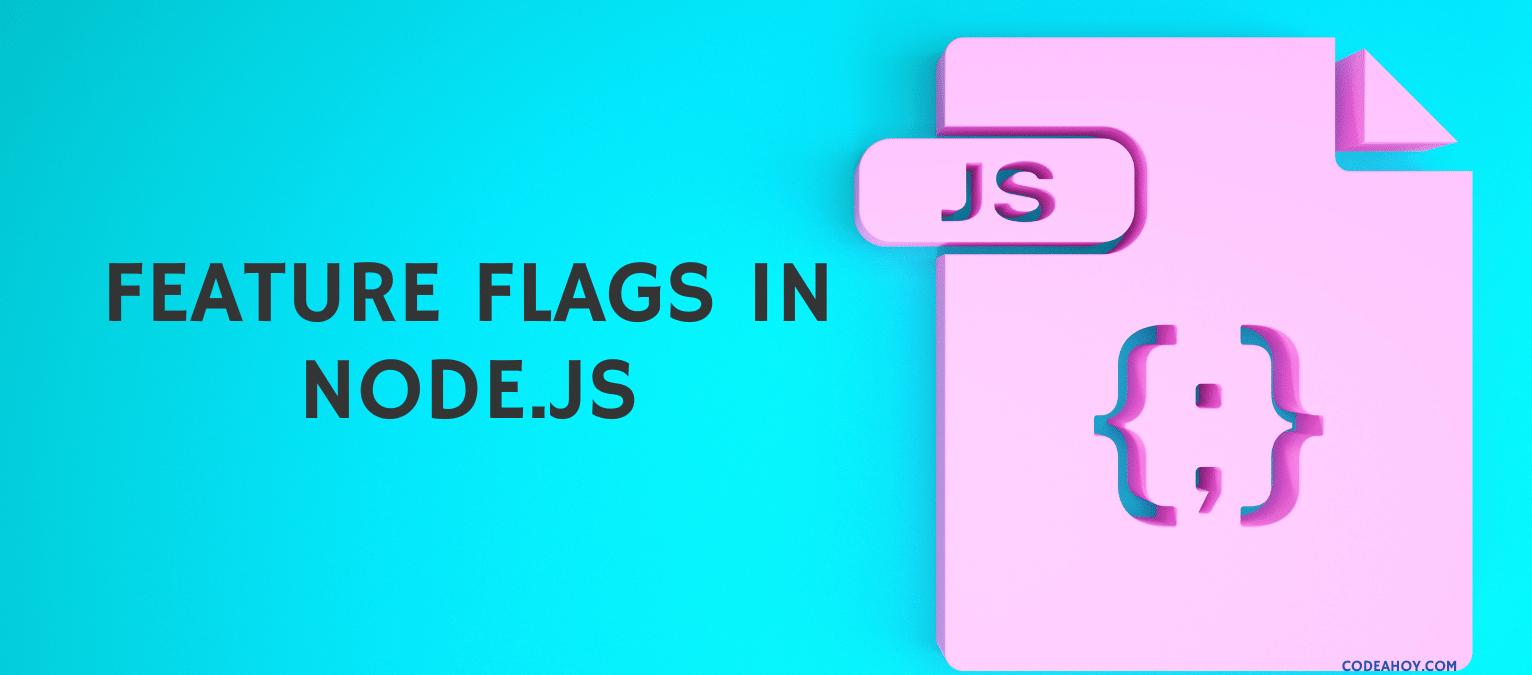
Feature flags (or feature toggles) is a powerful technique used by modern software teams to control the behavior of their code and features in production. Feature flags are used for:
- rolling new features out gradually
- branch by abstraction
- experimentation and testing
- migrations
- geographic restrictions
- permissions or kill switch
- testing in production
In this article, I’ll show you how to use feature flag in Node.js. For this example, let’s assume we have an e-commerce store and we’re implementing a new feature to change the sort order of the items because in our hypothetical example, the marketing team thinks changing the sort order will lead to more revenue. I’ll be using an open-source Node project called Crate which is an eCommerce subscription service for trendy clothes and accessories.
1. Put your feature behind feature flag in code
First, you’ll need to define the new feature in such a way it can be shown or hidden easily.
if featureFlag == "on" then
// show the new feature: new sort order
else
// show the old feature: old sort order
Once you have wrapped your code (new and old features) in a feature flag, you can easily control the behavior by changing the state of the feature flag or its targeting rules. If the flag is enabled (“on” variation,) we show the new feature. Otherwise, we don’t.
2. Create a feature flag
Next, create a new feature flag in Unlaunch that will control the feature you’re building. You can call it the “default-sort-order” or “crate-products”.
Targeting Users
A user is any object such as an email address, unique user id, a hash etc. to represent a unique user for which a feature flag is evaluated. Let’s define an internal user (engineering, QA or product team member) by email address to always show the “on” variation so they always get the new feature.
Targeting Rules
The rules that define which variation is evaluated are known as flag targeting rules. Here you can target by user attributes such as new users vs old, or by geo-location. Default Rule is the “catch-all” rule when targeting rules don’t match. In this case, the goal is to enable the feature for 10% of the users. To do that, we’ll define percentages under Default Rule.
3. Integrate Unlaunch Node.js SDK in your app
You’ll need to use the Unlaunch Node.js SDK to evaluate the feature flag you just defined from your app. If you’re using NPM, you can easily install the SDK:
npm install --save unlaunch-node-sdk
4. Call the feature flag and use variation to show or hide the feature
Open the file where wrapped the new feature in step #1. Let’s call the feature flag we just defined and use its result instead.
const UnlaunchFactory = require("unlaunch-node-sdk")
const variation = unlaunch.client.variation("crate-products", auth.user.email);
if(variation == 'on'){
return await models.Product.findAll({ order: [['id', 'ASC']] })
} else {
return await models.Product.findAll({ order: [['id', 'DESC']] })
}
Here’s the actual commit.
Here’s what the store looks like when the feature is disabled.
When you enable the feature, we can see the new sort order.
The cool thing is that now, we can enable or disable the feature on demand. We can roll it out to more users, kill it if it’s misbehaving, without changing the code or deploying new releases.
Summary
Feature flags are easy to set up and add a lot of flexibility to your application. Even if you use a feature flag service provider or create your own solution, I’m confident that your team will appreciate the idea of decoupling feature releases from code deployments because it removes a major source of stress.
GitHub Repo
Here’s the complete source code used in this blog post is available on GitHub. Here’s the commit with comments describing changes: https://github.com/Mahrukhizhar/crate/commits/main.
Learn More
- Blog post from Pete Hodgson at MartinFowler.com is a good read.
- Getting Started with Feature Flags
- Unlaunch Node.js SDK