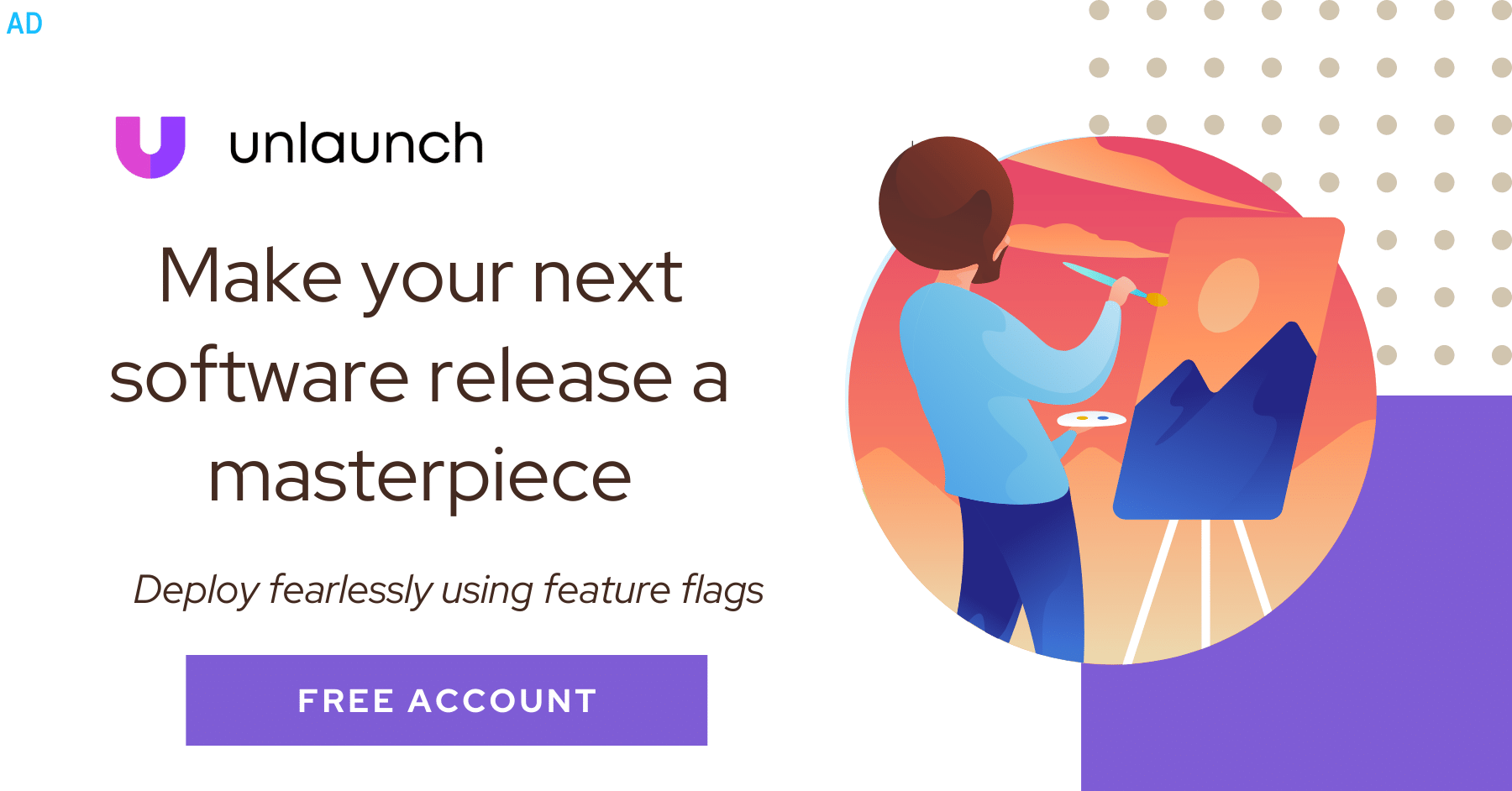
The Object.getClass()
method is used for getting the runtime class of an object.
String s = "foobar";
Class clazz = s.getClass();
System.out.println(clazz);
This will output:
class java.lang.String
When you call this method, it returns an object of type java.lang.Class
that represents the runtime class of the object the reference it pointing too. To understand this better, let’s take a look at an example. Suppose you have a method that accepts anything that implements the Queue
interface such as PriorityQueue
, ArrayDeque
, etc. In the code below, the getClass()
will return the actual class of the object that the reference object (Queue q
) is pointing to (rather than the class of the reference object, which isn’t very helpful.)
public static void main(String[] args) {
foo(new PriorityQueue());
foo(new ArrayDeque());
}
static void foo(Queue q) {
Class clazz = q.getClass();
System.out.println(clazz);
}
Output:
class java.util.PriorityQueue
class java.util.ArrayDeque
Notes:
- The
getClass()
method is most commonly used in reflection operations. - If you want the name of the class as a String, use
Object.getClass().getName()
method.
General Example
public class GetClassExample {
public static void main(String[] args) {
System.out.println("foo".getClass());
System.out.println((new ArrayList()).getClass());
System.out.println((new RuntimeException()).getClass());
List<String> l = new LinkedList<>();
System.out.println(l.getClass());
}
}
Output:
class java.lang.String
class java.util.ArrayList
class java.lang.RuntimeException
class java.util.LinkedList