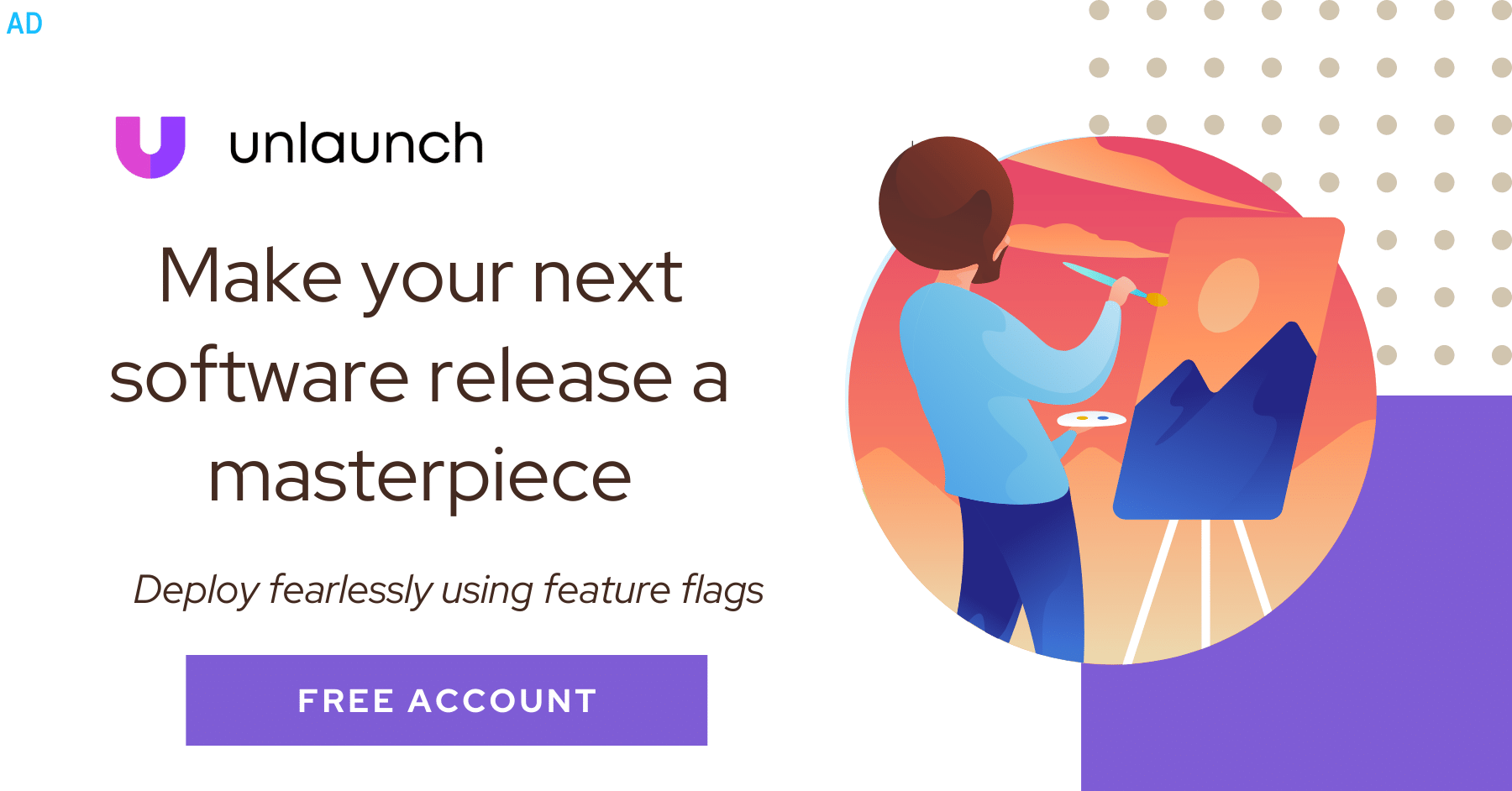
The java.lang.OutOfMemoryError
is a Java runtime error that happens when the Java Virtual Machine is unable to allocate an object owing to a lack of available memory in the Java heap. The Java Garbage Collection becomes unable to make space to accommodate a new object resulting in OutOfMemory
error being thrown.
To simulate OutOfMemoryError
, we could simply keep adding objects to a Java Collection such as LinkedList in a loop, until the JVM runs out of the heap memory and throws this error. In the example below, the infinite loop will add a new object of size 10MB on each iteration until it runs of memory.
public static void main(String[] args) {
List<byte[]> list = new LinkedList<>();
int index = 1;
while (true) {
byte[] b = new byte[10 * 1024 * 1024]; // 10MB byte object
list.add(b);
Runtime rt = Runtime.getRuntime();
System.out.printf("[%3s] Available heap memory: %s%n", index++, rt.freeMemory());
}
}
Output:
<truncated>
[358] Available heap memory: 163076576
[359] Available heap memory: 151542240
[360] Available heap memory: 140003280
[361] Available heap memory: 128468944
[362] Available heap memory: 116934608
[363] Available heap memory: 105399792
[364] Available heap memory: 93865456
[365] Available heap memory: 82331120
[366] Available heap memory: 70792160
[367] Available heap memory: 59257824
[368] Available heap memory: 47723488
[369] Available heap memory: 36186592
[370] Available heap memory: 24652256
[371] Available heap memory: 13466520
Exception in thread "main" java.lang.OutOfMemoryError: Java heap space
at SimulateOOM.main(SimulateOOM.java:11)