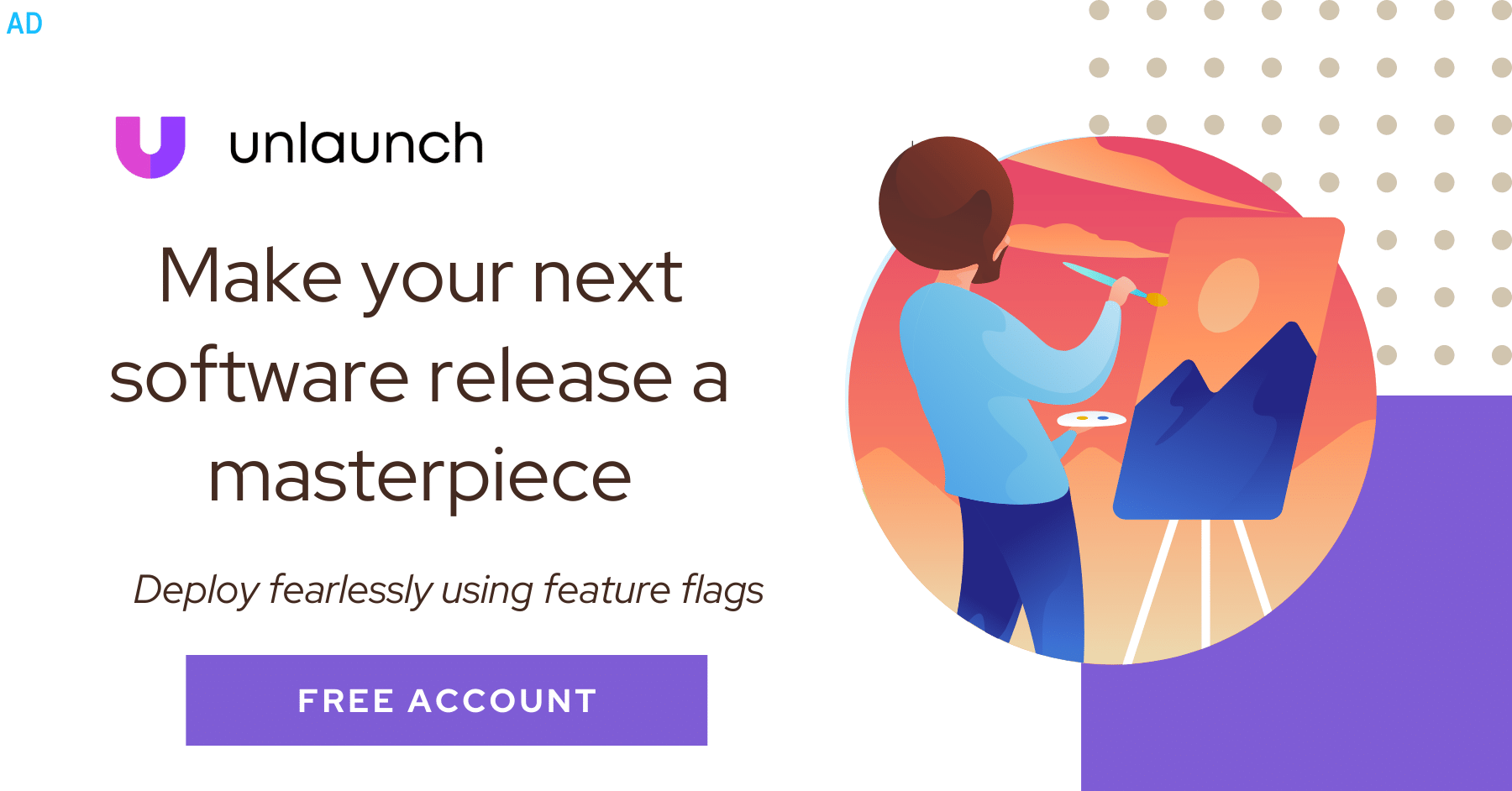
An array is a container object that is used for holding a fixed number of values of a single type. The size of the array is assigned when it is created.
In this tutorial, we’ll look at how to initialize and declare arrays in Java.
- Declaring Arrays in Java
- Array Initialization
- More Ways to Initialize Array Since Java 8
- Initializing 2d Arrays
- Other Examples
- Summary
Declaring Arrays in Java
We declare arrays in Java like any other variable: by providing a type and name.
// declare an array of integers
int[] numArray;
Each array declaration has two parts:
- The type of the array is indicated by
type[]
. Hereint[]
indicates that we are declaring an array of type integers - The name of the array. In the example above, the name of the array is
numArray
Note that the size of the array is not supplied. We’ll provide it later when initializing our array.
Similarly, you can declare arrays of other types:
double[] doubleArray;
String[] strArray;
SomeClass[] arr
=> An array holding objects ofSomeClass
.
Alternatively, you can also define the arrays as shown below by placing the brackets after the array’s name. However, prefer the first version as brackets right after the type makes it easier to identify.
int numArray[]; // valid declaraton type but its use is discouraged
Array Initialization
You can initialize an array in several ways:
Array Initialization Using the new
Operator
We can create arrays by using the new
operator and providing the size of the array.
int[] numArray = new int[5];
This creates a new array that holds up to 5 integers and is assigned to the numArray
variable. The array values are initialized using the default value of the data type it is holding. For our int[]
array, all (5) values will be initialized as 0. In the case of boolean[]
arrays, the values will be initialized to false
. To assign your own value, you can use the following syntax to assign a value at any index:
numArray[0] = 100; // initialize first element
Array initialization Using Shortcut
Another way to create and initialize an array is by using a shortcut and supplying the initial set of values when it is declared.
int[] numArray = {1, 2, 3, 5, 8};
The statement above creates an array of 5 elements - 1, 2, 3, 5 and 8. Note that you didn’t specify the size of the array. Java automatically creates an array based on the number of values you provide during initialization. For example, the array below will be of size 3 since we are providing 3 values during initialization:
int[] numArray = {1, 2, 3};
You can also optionally provide the new
keyword with initialization:
int[] numArray = new int[]{1, 2, 3, 5, 8};
Note that int[] numArray = {1, 2, 3}
doesn’t work with return
statements. If you have a method that’s returning a new array, this approach won’t work.
To recap, we learned the following ways of creating an array:
int[] numArray = new int[5];
int[] numArray = {1, 2, 3, 5, 8};
int[] numArray = new int[]{1, 2, 3};
More Ways to Initialize Array Since Java 8
IntStream.range(begin, end)
IntStream
is the int primitive specialization of Stream
class.
To declare and initialize an array of integers, you can also use the IntStream.range(begin, end)
. This method takes a range: begin
and end
as parameters and returns an IntStream
from beginning to end, where the end is non-inclusive. The resulting IntStream
can be then converted to an array using IntStream.toArray()
method.
// Create an array of size 10, initializing elements from 0 to 9
int [] numArray = IntStream.range(0, 10).toArray();
This creates an array of 10 elements, initializing elements from 0 to 9:
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
IntStream.rangeClosed(begin, end)
This is similar to the IntStream.range()
method but is inclusive of the end sequence that you specify. For example, if we create the same array as above using this method, such as:
// Create an array of size 11, initializing elements from 0 to 10
int [] numArray = IntStream.rangeClosed(0, 10).toArray();
This time we get an array to size 11, initialized from 0 to 10, because the end
sequence, 10 is inclusive. This gives us:
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
IntStream.of(int… values)
This gives us a sequential ordered stream (IntStream
) whose elements are the specified values. The order in which you specify the elements is preserved.
int [] myIntArray = IntStream.of(1, 3, 2, 8, 17, 6).toArray();
This gives us:
[1, 3, 2, 8, 17, 6]
To sort the values as the array is being initialized, you can use IntStream.sorted() method which returns a stream whose elements are in sorted order.
int [] myIntArray = IntStream.of(1, 3, 2, 8, 17, 6).sorted().toArray();
This gives us the sorted array, that is:
[1, 2, 3, 6, 8, 17]
Initializing 2d Arrays
Let’s look at how to declare and initialize multidimensional arrays in Java. The following statement creates a 2-dimensional, 10x5 array of ints.
int[][] numArray = new int[10][5];
or,
int[] numArray[] = new int[10][5];
To declare a 5x5 array and initialize it at the same time:
int[][] numArray=
{ {1,2,3,4,5}, {1,2,3,4,5}, {1,2,3,4,5}, {1,2,3,4,5}, {1,2,3,4,5} };
Other Examples
Create a char
Array in Java
To create a char array, you can use the same syntax that we have learned in this tutorial, that is,
char[] charArray = new char[5];
The statement above will array a char
array that can hold 5 characters and assigns it to the charArray
variable.
We can also convert String
to a char
array using String.toCharArray()
method:
String str = "jdk11";
char[] charArray = str.toCharArray();
This creates the following char
array:
['j', 'd', 'k', '1' , '1']
Summary
We looked at several ways to create and initialize an array in Java. Here’s a quick summary of all the ways we covered in this tutorial to declare and initialize arrays.
int[] numArray = new int[5]; // // [0, 0, 0, 0, 0]
int[] numArray = {1, 2, 3, 4, 5}; // [1, 2, 3, 4, 5]
int[] numArray = new int[]{1, 2, 3, 4, 5}; // [1, 2, 3, 4, 5]
// Using IntStream - Java 8
int [] numArray = IntStream.range(0, 100).toArray(); // [0, 1, ... 99]
int [] numArray = IntStream.rangeClosed(0, 100).toArray(); // [0, 1, ... 100]
int [] numArray = IntStream.of(5, 4, 3, 2, 1).toArray(); // [5, 4, 3, 2, 1]
int [] numArray = IntStream.of(5, 4, 3, 2, 1).sorted().toArray(); // [1, 2, 3, 4, 5]
// Multidimensional arrays
int[][] numArray = new int[10][5]; // 10x5 array initialized with 0's
int[] numArray[] = new int[10][5]; // Same as above
int[][] numArray={ {1,2,3,4,5}, {1,2,3,4,5}, {1,2,3,4,5}, {1,2,3,4,5}, {1,2,3,4,5} };