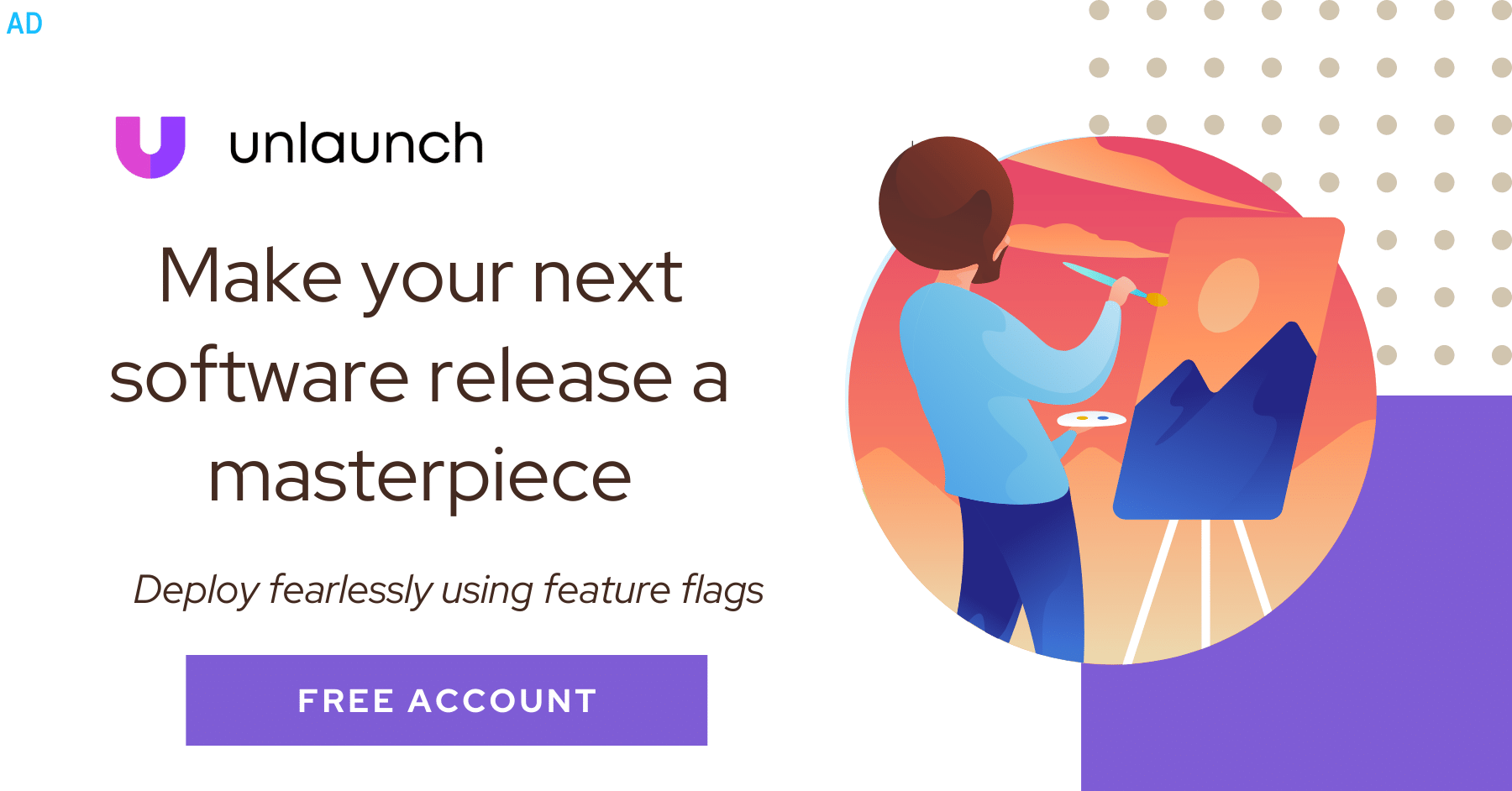
Monitoring and instrumenting applications in production is crucial. Not only it helps ensures that applications are running free of error and at desired performance levels, but also helps developers when they are troubleshooting an issue. Developers can connect to an application instance and do things like:
- retrieve the application health status,
- look at last few request traces,
- review audit logs,
- check various metrics such as average response times, etc.
- and more.
Monitoring applications in production is so critical that there are many tools and companies in this space. In this post, we’ll take a look at one such tool called Spring Boot Actuator.
- What is Spring Boot Actuator?
- How to Use Spring Boot Actuator
- Enabling and Exposing Endpoints
- Examples
- FAQs
What is Spring Boot Actuator?
Spring Boot provides many out of the box features to help developers monitor and manage their applications when they release it to production. One such feature is the Spring Boot Actuator. This module allows developers to expose operational information about their applications in production with minimum effort. This includes things like various metrics and counters, application health, request traces, interact with the application and more. This information can be accessed using HTTP or with JMX.
Definition of Actuator: An actuator is a manufacturing term that refers to a mechanical device for moving or controlling something. Actuators can generate a large amount of motion from a small change.
How to Use Spring Boot Actuator
Let’s walk through a complete example and see how to use Spring Boot Actuator in a project.
Adding Dependency
To use Actuator in your application, the very first thing you need to add its dependency, spring-boot-actuator
.
If you are using Maven, simply add the following to your pom.xml
under <dependencies>
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
For Gradle, use the following:
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-actuator'
}
Actuator Endpoints
By default, Actuator will automatically configure a bunch of HTTP endpoints under the prefix /actuator
such as /actuator/{id}
to let you monitor and interact with your applications. For example, the health endpoint provides basic application health information.
http GET "http://localhost:8080/actuator/health"
HTTP/1.1 200
Connection: keep-alive
Content-Type: application/vnd.spring-boot.actuator.v3+json
Date: Wed, 21 Oct 2020 18:11:35 GMT
Keep-Alive: timeout=60
Transfer-Encoding: chunked
{
"status": "UP"
}
Here’s a table showing some Actuator end-points and their brief description.
ID | Description |
---|---|
auditevents | Exposes audit events information for the current application. Requires an AuditEventRepository bean. |
beans | Displays a complete list of all the Spring beans in your application. |
caches | Exposes available caches. |
env | Exposes properties from Spring’s ConfigurableEnvironment. |
flyway | Shows any Flyway database migrations that have been applied. Requires one or more Flyway beans. |
health | Shows application health information. |
httptrace | Displays HTTP trace information (by default, the last 100 HTTP request-response exchanges). Requires an HttpTraceRepository bean. |
info | Displays arbitrary application info. |
loggers | Shows and modifies the configuration of loggers in the application. |
metrics | Shows “metrics” information for the current application. |
mappings | Displays a collated list of all @RequestMapping paths. |
quartz | Shows information about Quartz Scheduler jobs. |
scheduledtasks | Displays the scheduled tasks in your application. |
sessions | Allows retrieval and deletion of user sessions from a Spring Session-backed session store. Requires a servlet-based web application that uses Spring Session. |
shutdown | Lets the application be gracefully shutdown. Disabled by default. |
startup | Shows the startup steps data collected by the ApplicationStartup .Requires the SpringApplication to be configured with a BufferingApplicationStartup . |
threaddump | Performs a thread dump. |
Enabling and Exposing Endpoints
An endpoint is available when it is both enabled and exposed.
Enabling Endpoints
To enable an endpoint, use its management.endpoint.<id>.enabled
property. For example, to enable the shutdown
endpoint, add the following to your application.property
file:
management.endpoint.shutdown.enabled=true
If you want to disable all endpoints and pick and choose which ones you want to enable, set the following property to false
: management.endpoints.enabled-by-default=false
. The example below enables the info
endpoint and disables all other endpoints:
management.endpoints.enabled-by-default=false
management.endpoint.info.enabled=true
Since endpoints may contain sensitive information, not all endpoints are exposed by default on the Web. The following table shows the default exposure for the built-in endpoints:
ID | JMX | Web |
---|---|---|
auditevents | Yes | No |
beans | Yes | No |
caches | Yes | No |
conditions | Yes | No |
configprops | Yes | No |
env | Yes | No |
flyway | Yes | No |
health | Yes | Yes |
heapdump | N/A | No |
httptrace | Yes | No |
info | Yes | No |
logfile | N/A | No |
loggers | Yes | No |
metrics | Yes | No |
mappings | Yes | No |
quartz | Yes | No |
scheduledtasks | Yes | No |
sessions | Yes | No |
shutdown | Yes | No |
startup | Yes | No |
threaddump | Yes | No |
Exposing Endpoints
You can control which endpoints are exposed by using the include
and exclude
properties:
Property | Default |
---|---|
management.endpoints.jmx.exposure.exclude | |
management.endpoints.jmx.exposure.include | * |
management.endpoints.web.exposure.exclude | |
management.endpoints.web.exposure.include | health |
For example, to stop exposing all endpoints over JMX and only expose the health
and beans endpoints
, use the following property:
management.endpoints.jmx.exposure.include=health,beans
*
can be used to select all endpoints. For example, to expose everything over HTTP except the env
and beans
endpoints, use the following properties:
management.endpoints.web.exposure.include=*
management.endpoints.web.exposure.exclude=env,beans
Examples
1. Retrieving all Beans
The beans
endpoint provides information about the application’s beans. To retrieve the beans, make a GET request to /actuator/beans
:
curl 'http://localhost:8080/actuator/beans' -i -X GET
2. Retrieving all Caches
To retrieve the application’s caches, make a GET
request to /actuator/caches
:
curl 'http://localhost:8080/actuator/caches' -i -X GET
Sample Output:
{
"cacheManagers":{
"anotherCacheManager":{
"caches":{
"countries":{
"target":"java.util.concurrent.ConcurrentHashMap"
}
}
},
"cacheManager":{
"caches":{
"cities":{
"target":"java.util.concurrent.ConcurrentHashMap"
},
"countries":{
"target":"java.util.concurrent.ConcurrentHashMap"
}
}
}
}
}
3. Setting Log Level
To set the level of a logger, make a POST request to /actuator/loggers/{group.name}
with a JSON body that specifies the configured level for the logger group. The example below sets test
logger group to DEBUG
level.
$ curl 'http://localhost:8080/actuator/loggers/test' -i -X POST \
-H 'Content-Type: application/json' \
-d '{"configuredLevel":"debug"}'
For more examples, please see this document: Spring Boot Actuator Web API Documentation
FAQs
How can I change the default base path /actuator/{id}
path to something else say /monitor/{id}
?
The /actuator
base path can be configured by using the management.endpoints.web.base-path property
in your application.property
or application.yaml
file:
management.endpoints.web.base-path=/monitor
Now you can access actuator using /monitor/info
or /monitor/info
Attribution:
https://docs.spring.io/spring-boot/docs/current/reference/htmlsingle/#actuator