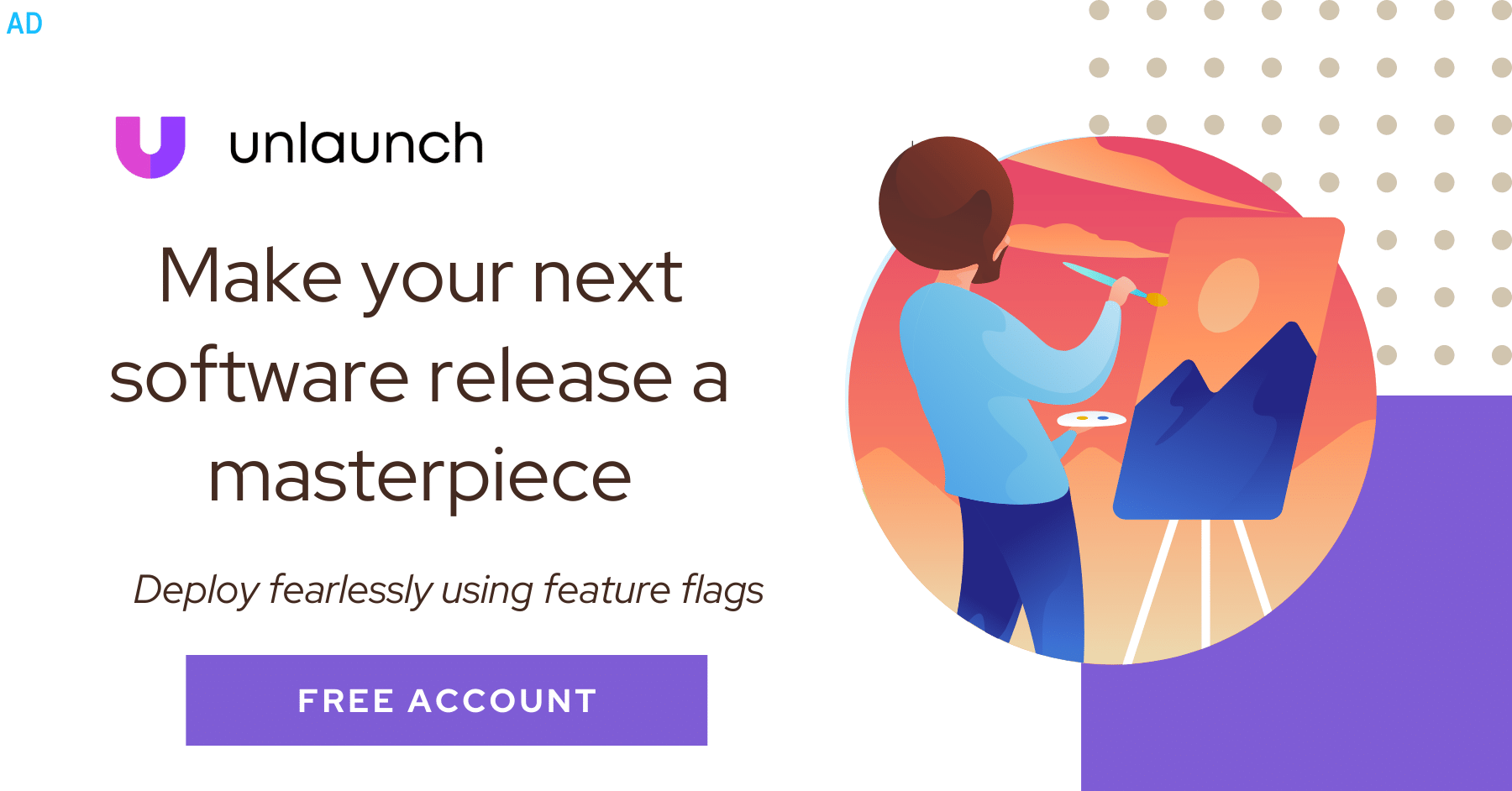
There are couple of ways to convert a numeric value represented by String
(e.g. “10”) into Integer
or int
type in Java. Let’s explore in this article with examples.
Integer.parseInt(…)
Integer.parseInt(String s) is the most common way of converting numbers represented by String variables to int
(primitive) in Java.
Integer.parseInt (…) Method Signature
public static int parseInt(String s)
throws NumberFormatException
Integer.parseInt(…) Examples
The example below shows how to use the parseInt(...)
method to convert a String
variable, s
into int
.
public static void main(String[] args) {
String s = "25";
int result = Integer.parseInt(s);
System.out.println(result);
}
Output:
25
Integer.parseInt(…) NumberFormatException
If you pass it an invalid value i.e. the string does not contain a parsable integer, or it is empty or null, Integer.parseInt(...)
will throw NumberFormatException.
public static void main(String[] args) {
String s = "abc";
int result = Integer.parseInt(s);
System.out.println(result);
}
Output:
Exception in thread "main" java.lang.NumberFormatException: For input string: "abc" at java.lang.NumberFormatException.forInputString(NumberFormatException.java:65) at java.lang.Integer.parseInt(Integer.java:580) at java.lang.Integer.parseInt(Integer.java:615) at com.example.demo.Main.main(Main.java:7)
Integer.valueOf(…)
There’s another method you can use to convert parsable strings to integer values in Java: Integer.valueOf(...)
. Just like parseInt(...)
, it is also a static method on the Integer
class. However, unlike parseInt(...)
, it returns a Integer
object (aka boxed primitive type). Use this if you want an Integer
object instead of the primitive int
.
Method Signature
The method definition is given below. It will also throw NumberFormatException
just like parseInt(...)
on invalid argument values.
public static Integer valueOf(String s)
throws NumberFormatException
Examples
public static void main(String[] args) {
String s = "25";
int result = Integer.valueOf(s);
System.out.println(result);
}
Output:
25
Apache Commons: NumberUtils.toInt(…)
If you’re using Apache Commons library, you can also use the NumberUtils.toInt(String str)
method to convert a parsable String
into int
. It differs from Integer.parseInt(...)
in that it returns 0
if the conversion fails due to string being invalid.