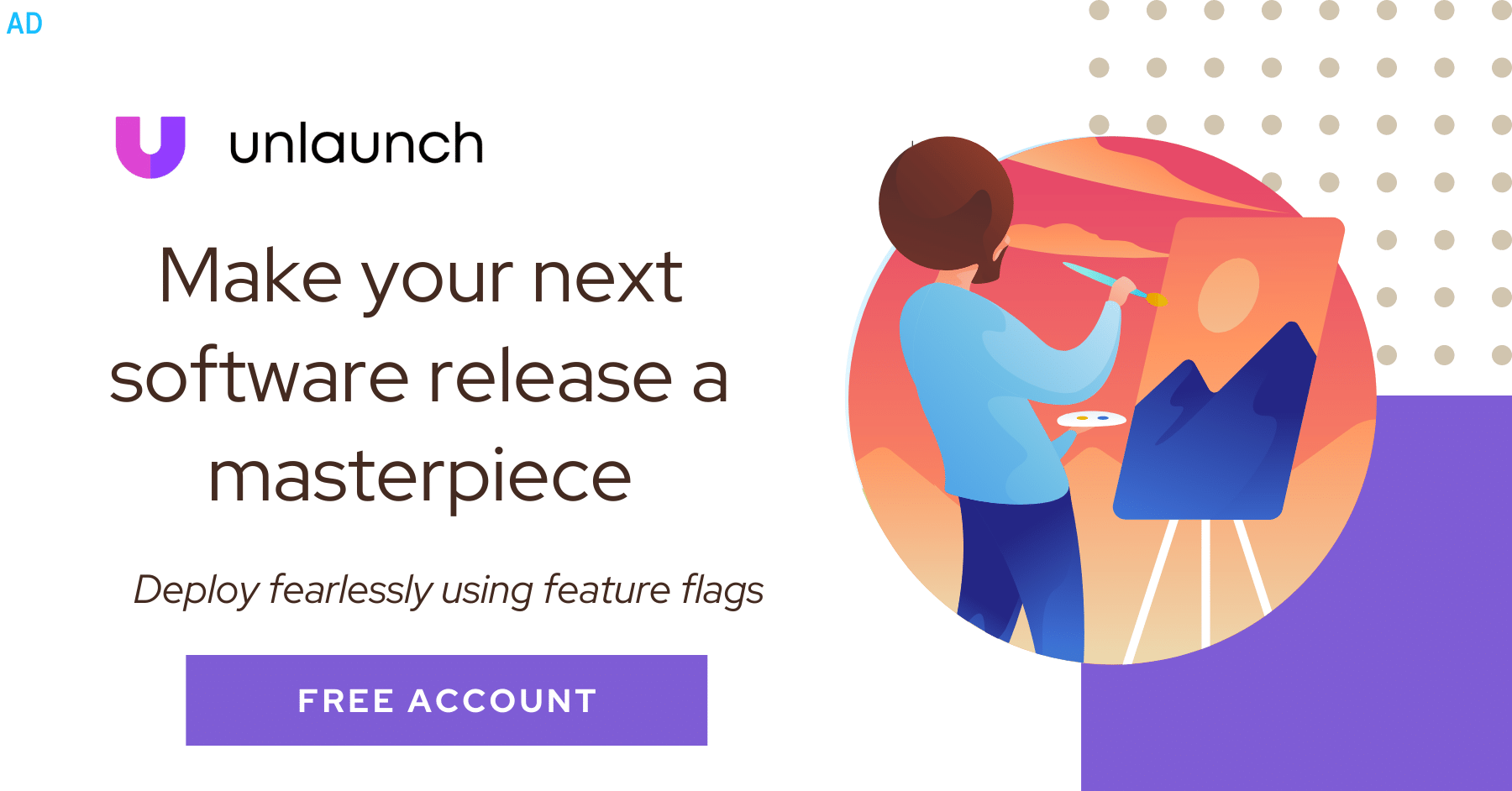
In this article, we’ll take a look at how to convert Streams to Arrays in Java.
The Streams API provides an easy to way to do the conversion using the built-in Stream.toArray(…) method. It takes a generator function as an argument to allocate the returned array and returns it to the caller. The generator function is an IntFunction which takes an integer argument and returns the allocated array.
1. Convert Stream to String[]
The following example shows how to convert a String stream to Sting[] using the concise representation of array constructor reference as the generator argument i.e. String[]::new
. You could also use lambda expression if you prefer i.e. size -> new String[size]
.
Examples
package com.codeahoy.ex;
import java.util.stream.Stream;
public class Main {
public static void main(String[] args) {
Stream<String> stringStream = Stream.of("Williams", "Ritchie",
"Knuth", "Thompson", "Kernighan", "Gosling");
String[] stringArray = stringStream.toArray(String[]::new);
for (String s: stringArray) {
System.out.println(s);
}
}
}
Output:
Williams Ritchie Knuth Thompson Kernighan Gosling
2. Convert Stream to Integer[]
To convert a Stream to Integer array, we’ll do something very similar to our last example, but pass in a new generator method Integer[]::new
to the toArray()
method.
Examples
package com.codeahoy.ex;
import java.util.stream.Stream;
public class Main {
public static void main(String[] args) {
Stream<Integer> integerStream = Stream.of(10, 20, 30, 40, 50);
Integer[] integerArray = integerStream.toArray(Integer[]::new);
for (Integer num: integerArray) {
System.out.println(num);
}
}
}
Output:
10 20 30 40 50
Things to Note
toArray(...)
is a terminal operation, that is, after this operation is applied, the stream is considered to be consumed and can not be used any further.toArray(...)
has a sibling method also calledtoArray(...)
but it doesn’t take any arguments. This method returns an array of type Object e.g.Object[]
.- If the type of the array returned from generator argument is not not a supertype of the of all the elements in the stream, an ArrayStoreException will be thrown. E.g. the code below will throw this exception because we’re trying to convert a stream of Doubles into Integer[].
public static void main(String[] args) {
Stream<Double> doubleStream = Stream.of(10.0, 20.0, 30.0);
// incompatible generator function
Integer[] integerArray = doubleStream.toArray(Integer[]::new);
}
Output:
Exception in thread "main" java.lang.ArrayStoreException: java.lang.Double at java.util.stream.Nodes$FixedNodeBuilder.accept(Nodes.java:1222) at java.util.Spliterators$ArraySpliterator.forEachRemaining(Spliterators.java:948) at java.util.stream.AbstractPipeline.copyInto(AbstractPipeline.java:481) at java.util.stream.AbstractPipeline.wrapAndCopyInto(AbstractPipeline.java:471) at java.util.stream.AbstractPipeline.evaluate(AbstractPipeline.java:545) at java.util.stream.AbstractPipeline.evaluateToArrayNode(AbstractPipeline.java:260) at java.util.stream.ReferencePipeline.toArray(ReferencePipeline.java:438) at com.codeahoy.ex.Main.main(Main.java:10)
If you want to learn more about Java Streams and how to use filter, map, reduce and sort functions, I’d encourage you to watch the following YouTube video.