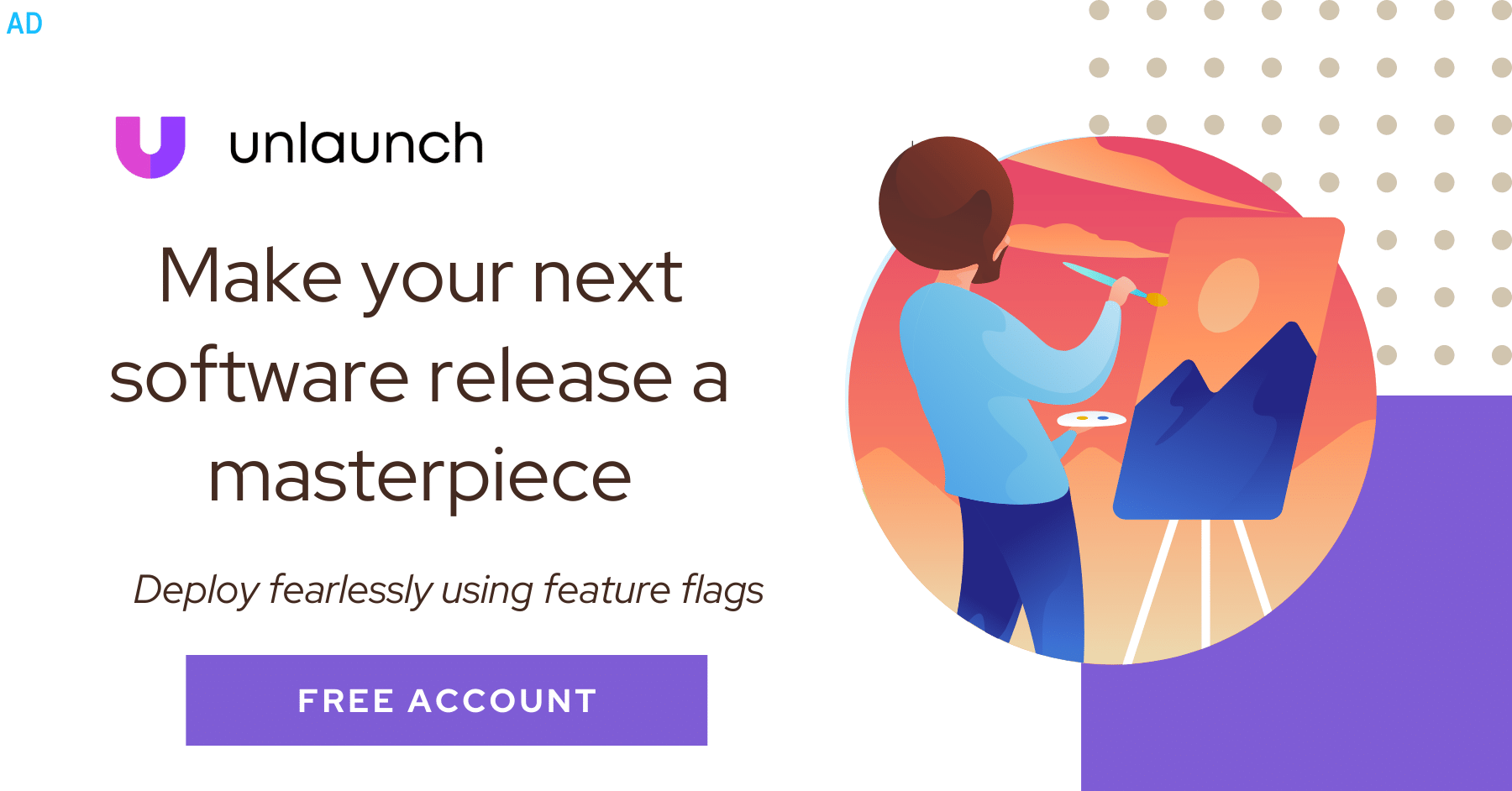
ArrayList is a resizable List implementation backed by an array. In other words, it implements the List interface and uses an array internally to support list operations such as add, remove, etc.
To convert ArrayList to array in Java, we can use the toArray(T[] a) method of the ArrayList class. It will return an array containing all of the elements in this list in the proper order (from first to last element.)
Here’s a short example to convert an ArrayList of integers, numbersList
, to int array.
Integer[] array = numbersList.toArray(new Integer[0]);
Replace Integer
with the type of ArrayList you’re trying to convert to array and it’ll work E.g. for String
:
String[] array = strList.toArray(new String[0]);
What’s with the weird-looking argument new Integer[0]
? The reason it is there because the type of returned array is determined using this argument. In other words, the toArray(...)
method uses the type of the argument, Integer
to create another array of the same type, places all elements from ArrayList into the array in order and returns it.
There is something else about the behavior of toArray(...)
method you must understand. Notice that we passed an empty array new Integer[0]
. This was intentional because if we pass a non-empty array and it has enough room to fit all elements, ArrayList will use this array instead of creating a new one. So by passing an empty array (size 0), we’re forcing ArrayList to create a new array and return it. The returned array is not connected to ArrayList in any way, but keep in mind that it is a shallow copy of the elements of the ArrayList.
Examples
Now let’s take a look at some examples.
- Covert ArrayList of integers to int array
- Covert ArrayList of strings to String array
- Alternate Way: The other toArray() method
- Convert ArrayList to Array Using Java 8 Streams
1. Covert ArrayList of integers to int array
In other words, ArrayList
package com.codeahoy.ex;
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Integer> list = new ArrayList<>();
// Add elements to the list
list.add(5);
list.add(10);
list.add(15);
// Convert ArrayList to Array
Integer[] array = list.toArray(new Integer[0]);
// Print the array
for (Integer n : array) {
System.out.println(n);
}
}
}
Output
5
10
15
2. Covert ArrayList of strings to String array
That is, ArrayList
package com.codeahoy.ex;
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
// Add elements to the list
list.add("List");
list.add("Set");
list.add("HashMap");
// Convert ArrayList to Array
String[] array = list.toArray(new String[0]);
// Print the array
for (String s : array) {
System.out.println(s);
}
}
}
Output:
List
Set
HashMap
3. Alternate Way: The other toArray() method
There’s another method, toArray() which doesn’t take any arguments. It behavior is identical to its overloaded cousin described above, except that it returns an array of objects i.e. Object []
. If you use this method, you need to cast it manually.
Object[] array = list.toArray();
4. Convert ArrayList to Array Using Java 8 Streams
We can use the toArray()
method of Streams API in Java 8 and above to convert ArrayList to array. I’m just putting it here for the sake of completeness. It requires converting ArrayList to a Stream first, which is unnecessary.
public static void main(String args[])
{
List<Integer> numList = new ArrayList<>();
numList.add(1);
Integer [] numArray = numList.stream().toArray( n -> new Integer[n]);
}
Java Versions Tested
The examples in this post were compiled and executed using Java 8. Everything contained in this post is accurate to all versions up to Java 13 (which is the latest version.)
The following video illustrates the difference between ArrayLists and Arrays, advantages and performance comparison of the two.
Comments (1)
Harry Henry Gebel
An array of Integers is not an array of ints.