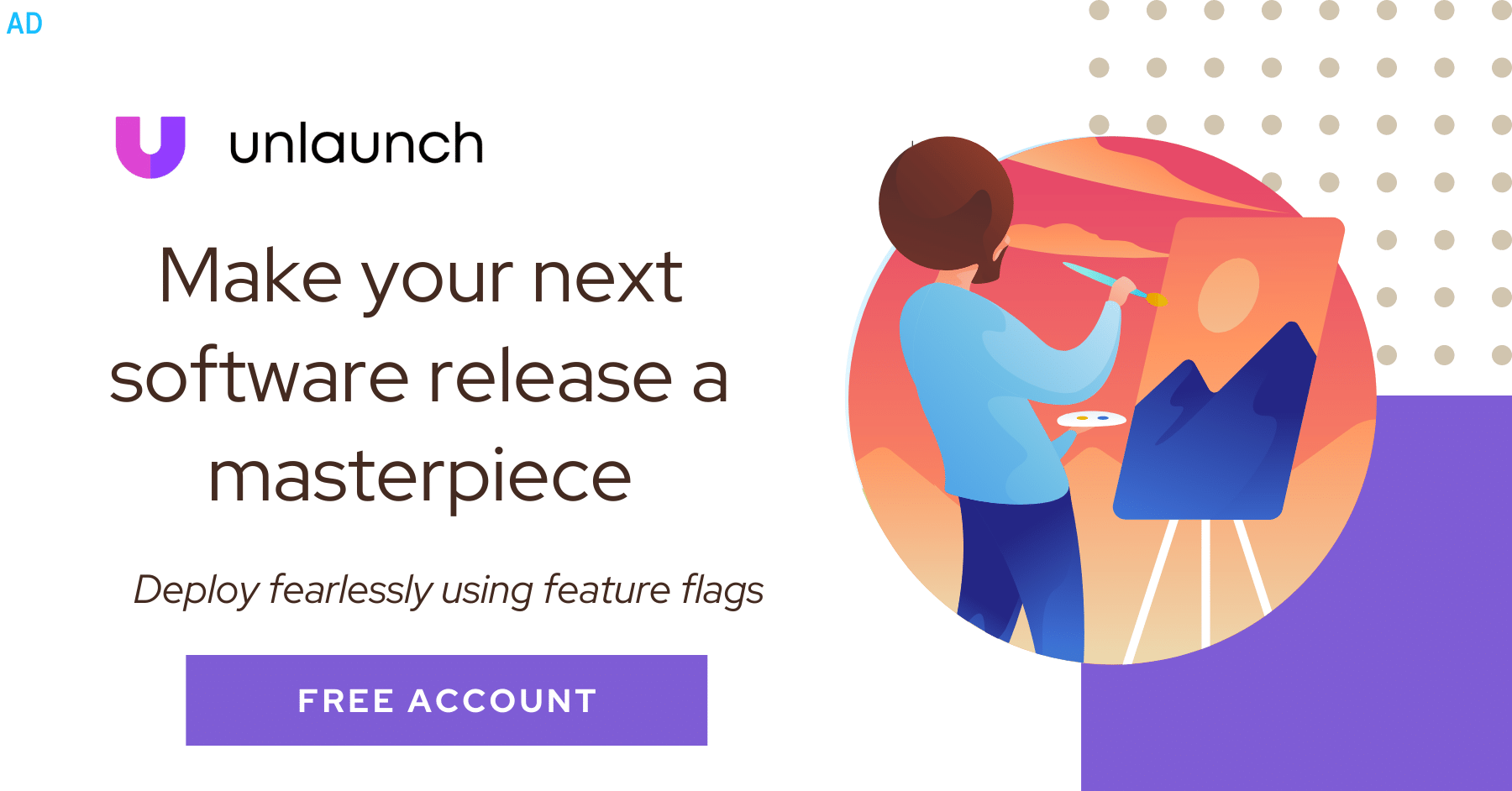
While strings are the preferred way of dealing with characters, character arrays (char []
)often make sense as buffers or in cases where working with arrays is more convenient. Side note: Read my warning about using the char data type.
Converting char arrays to strings is a pretty common use case. In this tutorial, we’ll look at how to convert character arrays to strngs using two different techniques.
1. Using String.valueOf(…) method
This is my preferred approach. It’s easy to remember because valueOf(...)
method is widely used to convert between types. In this case, String.valueOf(char [] data) takes a char [] array as an argument and returns its string representation. The returned string is “safe”: it can be modified and won’t affect the character array. Let’s look at an example.
package com.codeahoy.ex;
public class Main {
public static void main(String[] args) {
char[] charArray = { 'G', 'o', 'o', 'g', 'l', 'e' };
// Convert char [] to String using valueOf
String str = String.valueOf(charArray);
System.out.println(str);
}
}
Output
Google
There’s also another method called copyValueOf(char [] data) which is equivalent to valueOf(...)
. I think it’s there for backwards compatibility reasons and will advice that you prefer valueOf(...)
over it.
2. Using String class Constructor
String class in Java has a overloaded constructor which takes char [] array as argument. The String is created using the char [] array and is “safe”, that is, subsequent modification of the string or char [] array doesn’t affect each other. Let’s look at an example.
package com.codeahoy.ex;
public class Main {
public static void main(String[] args) {
char[] charArray = { 'C', 'o', 'd', 'e', 'A', 'h', 'o', 'y' };
// Convert char [] to String
String str = new String(charArray);
System.out.println(str);
}
}
Output
CodeAhoy
There are other ways of converting character array to string in Java but these two are the ones that are most commonly used in practice. Until next time.