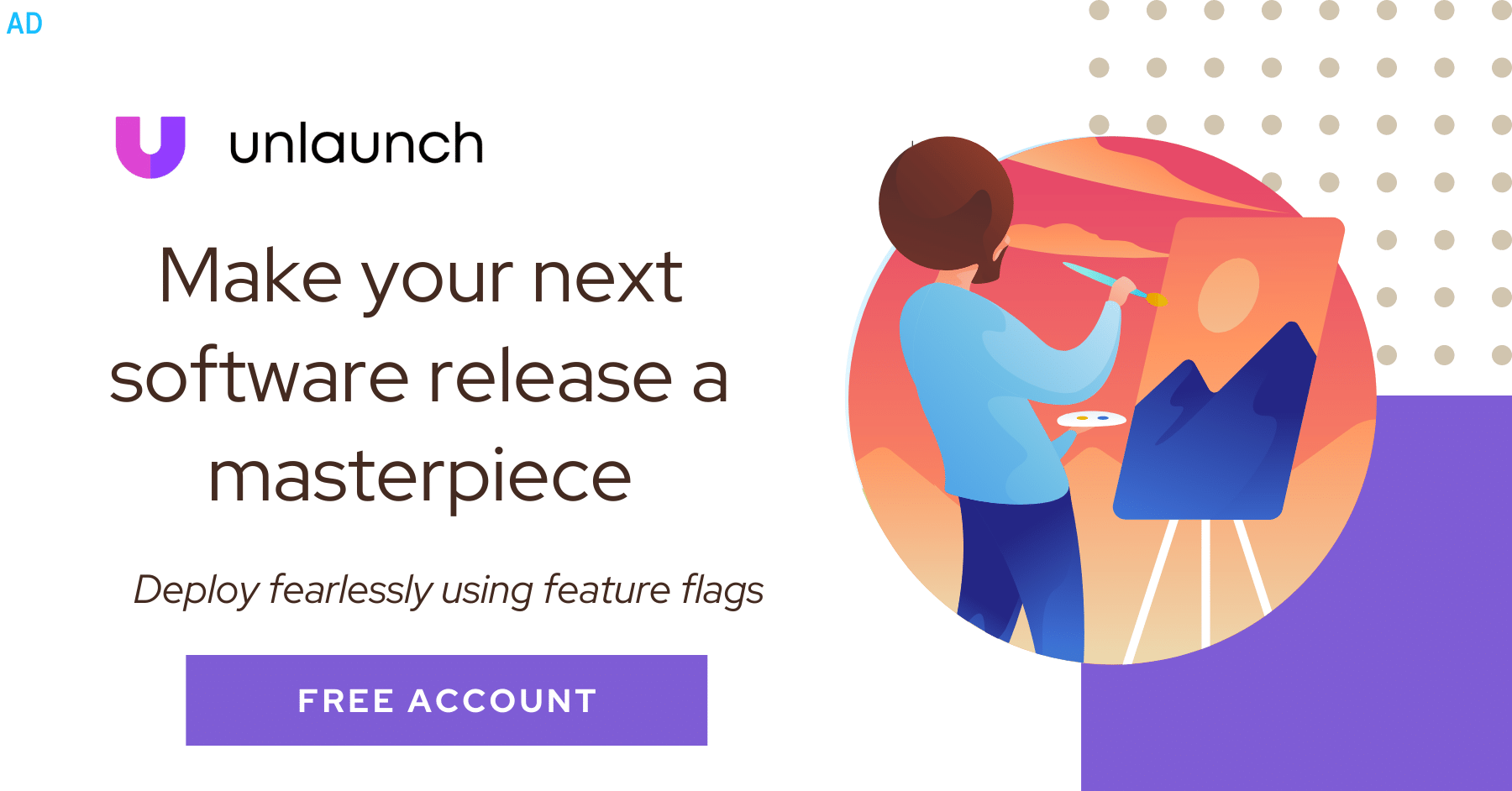
There are a couple ways we can convert a string into an array of characters in Java. The technique you’d use depends on your use case. Let’s take a look at the available options.
- Using String.toCharArray() method - Converts the whole String
- Using String.getChars(…) method - Converts only a subset of String
1. Using String.toCharArray() method
You can use the toCharArray() method of the String
class to convert it to an array i.e. char[]
. When you call this method, it returns a new character array corresponding to the string. Use this method when you want the entire string to be converted to a character array e.g. if a method in an older library requires that you pass it character array and you have a string. Let’s take a look at an example.
package com.codeahoy.ex;
public class Main {
public static void main(String[] args) {
String str = "CodeAhoy";
// Convert String to char array
char [] charArray = str.toCharArray();
System.out.println("Length of charArray: "
+ charArray.length);
for (char c: charArray) {
System.out.println(c);
}
}
}
Output
Length of charArray: 8
C
o
d
e
A
h
o
y
2. Using String.getChars(…) method
There’s another, slightly more involved, way of converting a string to an array of characters: String.getChars(…). Let’s take a look at JavaDocs:
public void getChars(int srcBegin,
int srcEnd,
char[] dst,
int dstBegin)
It copies characters of a string from srcBegin
to srcEnd
to the dst
char array starting at index dstBegin
. This method is useful if you want to convert only a subset of string to character array, instead of the whole thing.
package com.codeahoy.ex;
public class Main {
public static void main(String[] args) {
String str = "CodeAhoy Articles on Java";
// Create array big enough to fit 'CodeAhoy'
char[] destArray = new char[8];
// Copy 'CodeAhoy' from string to char array
str.getChars(0, 8, destArray, 0);
System.out.println(destArray);
}
}
That’s all. We looked at two way of converting a String
to char[] in Java. Please keep in mind that if you just need a single letter from a string as character, you can use the charAt(index) method which will return the char
value at the specified position in the String, that is,
String str = "CodeAhoy";
System.out.println(str.charAt(4)); // Prints 'A'
If you’re interested, the following video is a good introducion to the char
type in Java. See you next time!