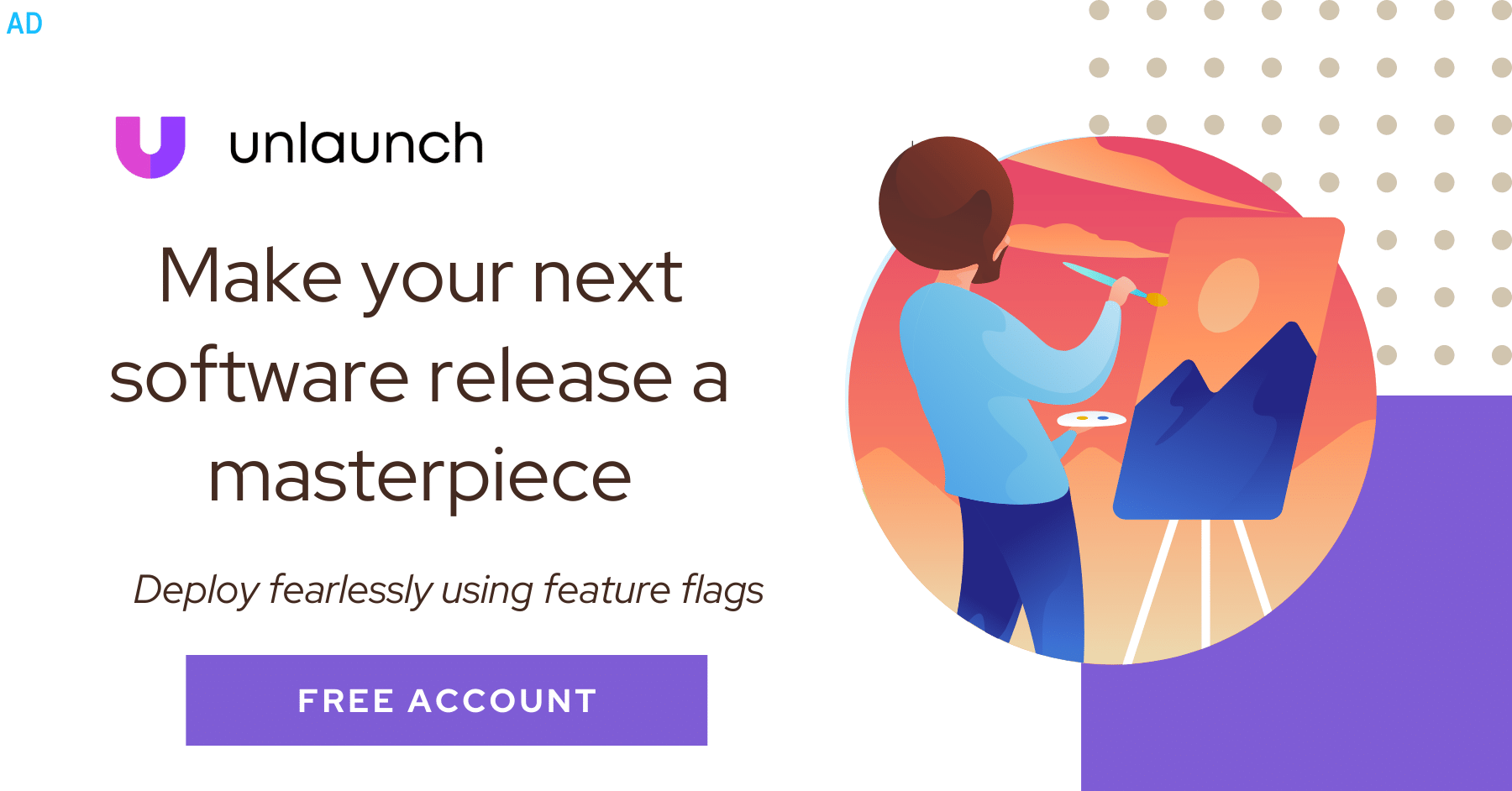
An array is a container that holds a fixed number of values. It requires all values to be of the same type. For example, all elements must be of type int
or double
but not both int
and double
. Each item in the array is called an element and is accessed by a numberical index which starts at 0. The length of an array is the number of elements that the array contains. The length is defined when the array is created and is fixed after creation.
To find length of an array in Java, you can can use the built-in length
field of an array. This field is available for all array data types (e.g. int[]
, char[]
, etc.) and returns the number of items in the array.
As an example, if you have an array of int[]
, you can find its length (number of elements) by using the length
field of the array.
int[] arrNumbers = {1,2,3,4};
int length = arrNumbers.length;
System.out.println("length is: " + length);
The code above will print 4 (output below) because there are 4 elements in array.
length is: 4
Other Notes
- The
length
variable is declared as final because the length of an array is defined only when it is created and cannot be changed later. You can safely use thelength
field everywhere without worrying about changing it inadvertently. length
vssize()
:size()
is a method which is defined in java.util.Collection class. It performs the same function for collections aslength
does for arrays: returns the number of elements.length
vsString.length()
: Developers often mix these up.String.length()
is a method which returns the number of characters in the string. (Food for thought: Internally, strings are backed bychar[]
arrays so perhaps they aren’t not so different 🤔)
Here’s the complete code example for this post.
public class ArrayLength {
public static void main(String[] args) {
// Let's define some arrays
int[] arrNumbers = {1,2,3,4};
char[] arrCharacters = {'a', 'b', 'c'};
String[] arrStrings = {"java", "c++", "c", "react", "spring"};
Object[] arrObj = new Object[10]; // new array with uninitialized elements
// Print length of arrays
System.out.println("arrNumbers.length is: " + arrNumbers.length);
System.out.println("arrCharacters.length is: " + arrCharacters.length);
System.out.println("arrStrings.length is: " + arrStrings.length);
System.out.println("arrObj.length is: " + arrObj.length);
}
}
Output
arrNumbers.length is: 4
arrCharacters.length is: 3
arrStrings.length is: 5
arrObj.length is: 10