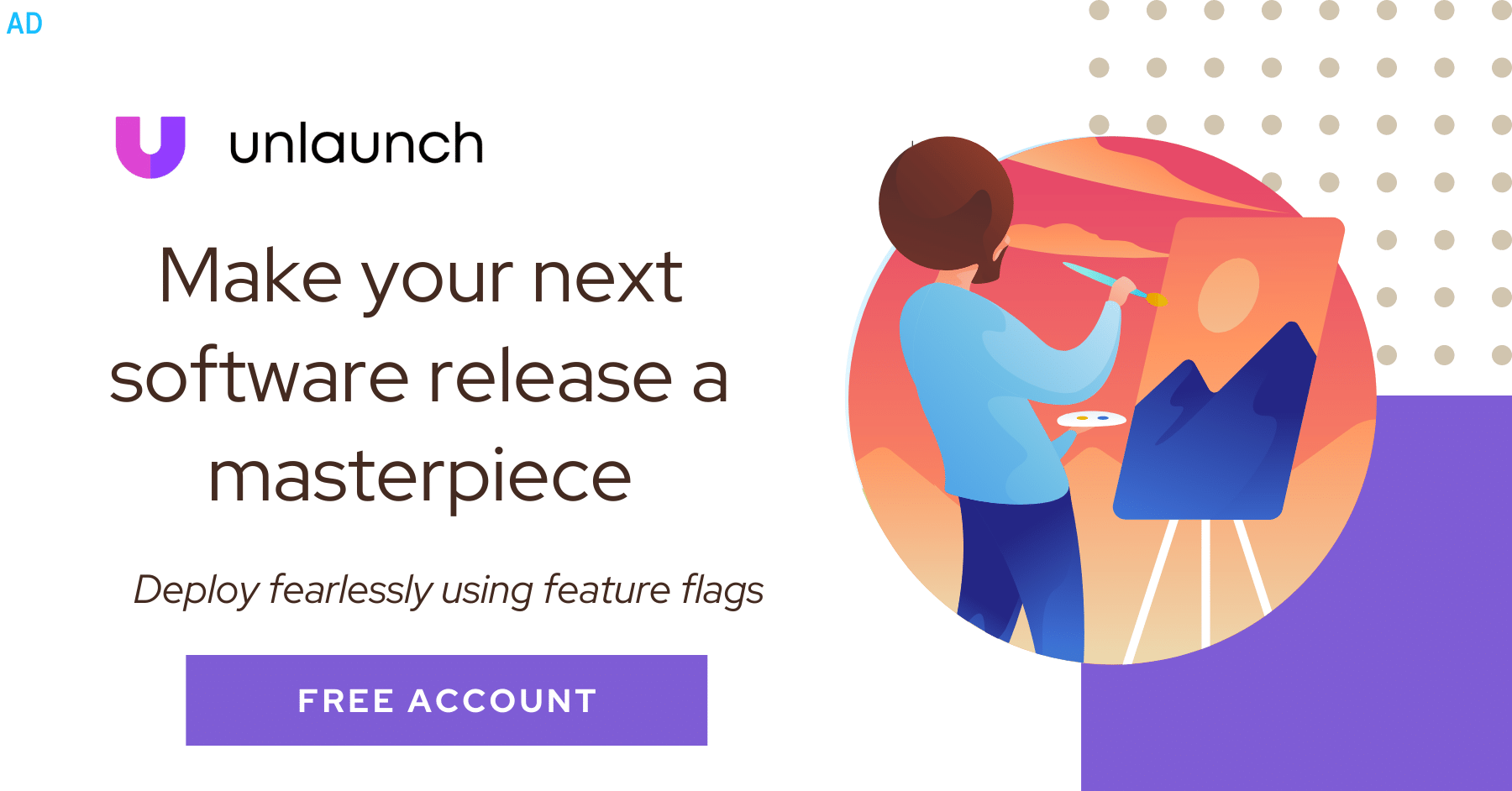
Apache Tomcat and Eclipse Jetty are two of the most popular web servers and Java Servlet containers. Tomcat is more widely used compared to Jetty and has significantly more market share. On the other hand, Jetty is light-weight, more compact and has a smaller CPU and memory footprint. For this reason, it is easier to work with it in development than Tomcat. This is not to suggest that Jetty isn’t good for production - in my experience, it is as performant as Tomcat if not more.
Many developers prefer Jetty over Tomcat during the development stage when they want to rapidly launch and test web apps on their local machines. Spring Boot web starter uses Tomcat as the default embedded server. Let’s take a look at how to change it to Jetty.
If you’d like to change the embedded web server to Jetty in a new Spring Boot web starter project, you’ll have to:
- Exclude Tomcat from web starter dependency, since it is added by default
- Add the Jetty dependency
Step 1: Exclude Tomcat
Find the following dependency in pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
Replace it with:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
Step 2: Add Jetty
Add the following dependency to your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jetty</artifactId>
</dependency>
Please note that there are some other starters e.g. ThymeLeaf templating engine, etc. that might pull in Tomcat by default. If you’re using one of these, you’ll have to manually exclude Tomcat from all such dependencies. When I’m running into issues, I look at maven dependencies to check if Tomcat is being pulled in. You could check for this by either inspecting the dependencies on command line e.g. mvn dependency:tree -Dverbose
or using the inspector in your favourite IDE e.g. IntelliJ or Eclipse.
GitHub Project
I created a SpringBoot Jetty Starter project on GitHub which excludes Tomcat and uses Jetty as the web server. Here’s the complete pom file which shows how this is done.
To run the project, you’ll need to clone it first.
git clone https://github.com/codeahoy/BootWebJetty.git
To run it,
mvn clean package spring-boot:run
In the output, you should see Jetty running on port 8080.
Jetty started on port(s) 8080 (http/1.1) with context path '/'
I created a sample controller called greeting, so you should be able to open http://127.0.0.1:8080/greeting and see a message printed to the browser window.
The project uses SpringBoot 2.2.4 and Java 8. You can change this in the pom file if you want to use different versions.
That’s all. If you found this post useful, please share it using the sharing buttons below. It will help us grow. Thank you.