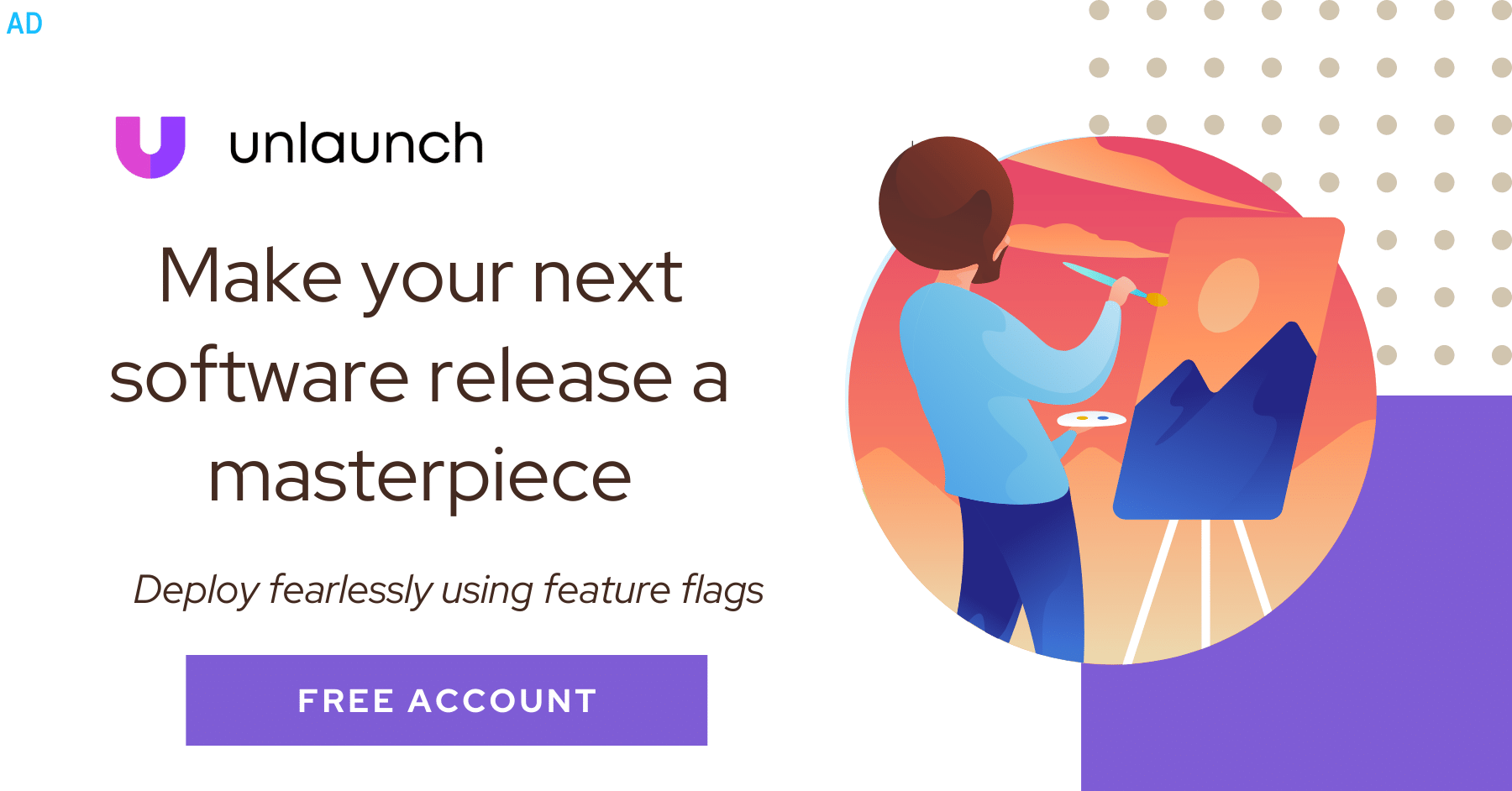
Spring Boot web applications use embedded Tomcat as the webserver. By default, Tomcat uses HTTP port 8080 to deploy web applications. Let’s see how to change the default port of Tomcat. We can configure the port in a few different ways.
- Change the Port Using Configuration Files
- Command Line Argument
- Spring Boot 2: Change Port Using the ConfigurableServletWebServerFactory Class
- Spring Boot 1: Change Port Using the ConfigurableEmbeddedServletContainer
- Use a Random HTTP Port
Change the Port Using Configuration Files
You can easily set the HTTP port for Tomcat be setting the server.port
variable to the desired port in the Spring Boot configuration file. This is the easiest and preferred way of configuring the port.
In your application.properties file,
/src/main/resources/application.properties
server.port=8000
If you use YAML file for your cofiguration,
/src/main/resources/application.yml
server:
port: 8000
Command Line Argument
Alternatively, you can set the SERVER_PORT
variable in your OS environment or pass it on the command line:
java -jar -Dserver.port=8000 spring-boot-app-1.0.jar
Spring Boot 2: Change Port Using the ConfigurableServletWebServerFactory Class
In Spring Boot 2, you can use the ConfigurableServletWebServerFactory config to configure the HTTP port. Please note that this example will not work in Spring Boot 1.x. See the next section.
public class CustomContainer implements
WebServerFactoryCustomizer<ConfigurableServletWebServerFactory> {
public void customize(ConfigurableServletWebServerFactory factory) {
factory.setPort(8000);
}
}
Spring Boot 1: Change Port Using the ConfigurableEmbeddedServletContainer
If you’re using Spring Boot 1.x, you can use the EmbeddedServletContainerCustomizer
class to configure the HTTP port.
@Component
public class CustomContainer implements EmbeddedServletContainerCustomizer {
@Override
public void customize(ConfigurableEmbeddedServletContainer container) {
container.setPort(8000);
}
}
Use a Random HTTP Port
If you wish Spring Boot to pick a random HTTP port, which is useful if you want to avoid clashes, you can do this by setting server.port=0
. By setting server.port
to 0, Spring Boot will scan your machine and use an available port.
That’s all. I’m sure there are more ways of changing the default port in Tomcat. My preferred approach is to set server.port
in the configuration file because it’s the easiest and most convenient.
If you know other ways of changing ports in Tomcat, please leave a comment below and share. Thanks.
Comments (1)
camel
BTW: @SpringBootApplication public class CustomApplication { public static void main(String[] args) { SpringApplication app = new SpringApplication(CustomApplication.class); app.setDefaultProperties(Collections .singletonMap(“server.port”, “9090”)); app.run(args); } }